创建progressbar 通知栏
时间: 2024-09-13 08:10:58 浏览: 37
在Android中,创建进度条通知栏通常是在服务或广播接收者中完成的,因为它们可以在后台运行而不显示完整的Activity。下面是一个简单的示例,展示如何在一个服务中使用NotificationCompat.Builder来创建一个包含进度条的通知:
首先,在你的服务或BroadcastReceiver中添加权限:
```java
<uses-permission android:name="android.permission.VIBRATE" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
```
然后创建一个Notification实例:
```java
import androidx.core.app.NotificationCompat;
import androidx.core.app.NotificationManagerCompat;
public class MyService extends Service {
private NotificationManagerCompat notificationManager;
private int progressId = R.drawable.ic_notification_progress; // 设置进度条的图标
@Override
public void onCreate() {
super.onCreate();
notificationManager = NotificationManagerCompat.from(this);
createProgressNotification();
}
private void createProgressNotification() {
int notificationId = 1;
NotificationCompat.Builder builder = new NotificationCompat.Builder(this)
.setContentTitle("我的任务")
.setContentText("正在处理...")
.setSmallIcon(R.mipmap.ic_launcher)
.setProgress(100, 0, false) // 进度条最大值、初始值和是否循环
.setVisibility(NotificationCompat.VISIBILITY_PUBLIC)
.setOngoing(true); // 表示通知还在继续
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
builder.setProgressStyle(NotificationCompat.Style.indeterminateHorizontalProgressStyle());
} else {
builder.setProgressStyle(new NotificationCompat.InboxStyle()
.bigContentTitle("进度")
.bigText("0%"));
}
Intent intent = new Intent(this, MainActivity.class); // 点击通知跳转到主界面
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intent, 0);
builder.setContentIntent(pendingIntent);
builder.setProgressPercent(50); // 更新进度条百分比
notificationManager.notify(notificationId, builder.build());
// 模拟更新进度
Handler handler = new Handler();
handler.postDelayed(() -> {
updateProgress();
handler.postDelayed(updateProgress, 1000);
}, 1000);
}
private void updateProgress() {
int currentProgress = ... // 根据实际工作进度计算当前进度
if (currentProgress >= 100) {
stopSelf(); // 任务完成后停止服务
} else {
NotificationCompat.Builder builder = (NotificationCompat.Builder) notificationManager.getNotificationById(progressId).copy();
builder.setProgressPercent(currentProgress);
notificationManager.notify(progressId, builder.build());
}
}
}
```
在这个例子中,我们每隔一秒更新一次进度,并在进度达到100%时关闭通知。请注意,你需要根据实际应用需求调整这部分逻辑。
阅读全文
相关推荐
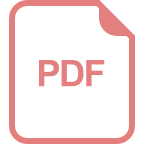
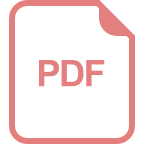
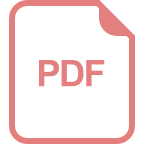
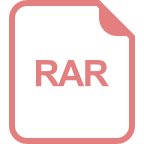
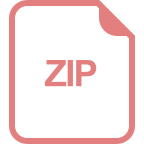
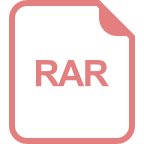
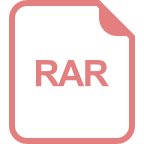
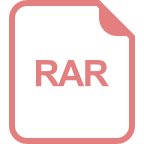
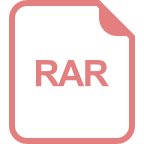
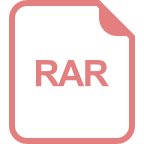
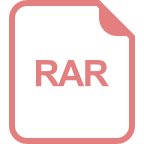
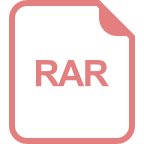
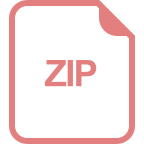
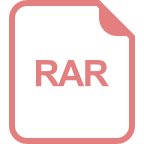
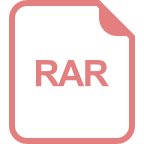
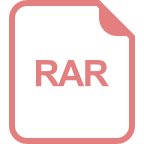
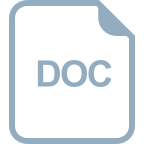
