首页method = clazz.getDeclaredMethod(methodName); java 后端 method.invoke调用方法如何传参
method = clazz.getDeclaredMethod(methodName); java 后端 method.invoke调用方法如何传参
时间: 2024-09-28 17:05:56 浏览: 29
`method.invoke()` 是 Java 中用于动态调用对象方法的一个方法,它通常与反射(Reflection)一起使用。当你通过 `getDeclaredMethod()` 获取到一个方法对象后,你可以使用这个方法来在特定的对象上调用该方法。
传参的过程如下:
```java
Object obj = ...; // 要操作的对象
Object[] args = {arg1, arg2, ...}; // 方法需要的参数列表
// 确保方法可以接受提供的参数
if (method.getParameterCount() == args.length) {
try {
method.invoke(obj, args); // 使用obj作为目标对象,并将args数组传入
} catch (IllegalAccessException | IllegalArgumentException | InvocationTargetException e) {
e.printStackTrace(); // 处理可能出现的异常
}
} else {
System.out.println("参数数量不匹配!");
}
```
这里,`getParameterCount()` 用于获取方法需要的参数个数,`invoke()` 方法会尝试将 `args` 数组中的值依次对应地传递给方法。如果存在权限问题、参数类型错误或者方法抛出异常,你需要捕获并处理相应的异常。
阅读全文
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
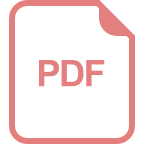
java反射获取和调用方法
在上面的代码中,我们首先获取了 `Person` 类的 `Class` 对象,然后通过 `getDeclaredMethod` 方法获取了 `getName` 方法,最后通过 `invoke` 方法调用了该方法,并传入了 `"zhangsan"` 作为参数。 三、多个参数的...
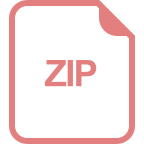
java项目,课程设计-ssm病人跟踪治疗信息管理系统
病人跟踪治疗信息管理系统采用B/S模式,促进了病人跟踪治疗信息管理系统的安全、快捷、高效的发展。传统的管理模式还处于手工处理阶段,管理效率极低,随着病人的不断增多,传统基于手工管理模式已经无法满足当前病人需求,随着信息化时代的到来,使得病人跟踪治疗信息管理系统的开发成了必然。
本网站系统使用动态网页开发SSM框架,Java作为系统的开发语言,MySQL作为后台数据库。设计开发了具有管理员;首页、个人中心、病人管理、病例采集管理、预约管理、医生管理、上传核酸检测报告管理、上传行动轨迹管理、分类管理、病人治疗状况管理、留言板管理、系统管理,病人;首页、个人中心、病例采集管理、预约管理、医生管理、上传核酸检测报告管理、上传行动轨迹管理、病人治疗状况管理,前台首页;首页、医生、医疗资讯、留言反馈、个人中心、后台管理、在线咨询等功能的病人跟踪治疗信息管理系统。在设计过程中,充分保证了系统代码的良好可读性、实用性、易扩展性、通用性、便于后期维护、操作方便以及页面简洁等特点。
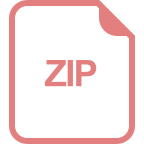
黑板风格计算机毕业答辩PPT模板下载
资源摘要信息:"创意经典黑板风格毕业答辩论文课题报告动态ppt模板"
在当前数字化教学与展示需求日益增长的背景下,PPT模板成为了表达和呈现学术成果及教学内容的重要工具。特别针对计算机专业的学生而言,毕业设计的答辩PPT不仅仅是一个展示的平台,更是其设计能力、逻辑思维和审美观的综合体现。因此,一个恰当且创意十足的PPT模板显得尤为重要。
本资源名为“创意经典黑板风格毕业答辩论文课题报告动态ppt模板”,这表明该模板具有以下特点:
1. **创意设计**:模板采用了“黑板风格”的设计元素,这种风格通常模拟传统的黑板书写效果,能够营造一种亲近、随性的学术氛围。该风格的模板能够帮助展示者更容易地吸引观众的注意力,并引发共鸣。
2. **适应性强**:标题表明这是一个毕业答辩用的模板,它适用于计算机专业及其他相关专业的学生用于毕业设计课题的汇报。模板中设计的版式和内容布局应该是灵活多变的,以适应不同课题的展示需求。
3. **动态效果**:动态效果能够使演示内容更富吸引力,模板可能包含了多种动态过渡效果、动画效果等,使得展示过程生动且充满趣味性,有助于突出重点并维持观众的兴趣。
4. **专业性质**:由于是毕业设计用的模板,因此该模板在设计时应充分考虑了计算机专业的特点,可能包括相关的图表、代码展示、流程图、数据可视化等元素,以帮助学生更好地展示其研究成果和技术细节。
5. **易于编辑**:一个良好的模板应具备易于编辑的特性,这样使用者才能根据自己的需要进行调整,比如替换文本、修改颜色主题、更改图片和图表等,以确保最终展示的个性和专业性。
结合以上特点,模板的使用场景可以包括但不限于以下几种:
- 计算机科学与技术专业的学生毕业设计汇报。
- 计算机工程与应用专业的学生论文展示。
- 软件工程或信息技术专业的学生课题研究成果展示。
- 任何需要进行学术成果汇报的场合,比如研讨会议、学术交流会等。
对于计算机专业的学生来说,毕业设计不仅仅是完成一个课题,更重要的是通过这个过程学会如何系统地整理和表述自己的思想。因此,一份好的PPT模板能够帮助他们更好地完成这个任务,同时也能够展现出他们的专业素养和对细节的关注。
此外,考虑到模板是一个压缩文件包(.zip格式),用户在使用前需要解压缩,解压缩后得到的文件为“创意经典黑板风格毕业答辩论文课题报告动态ppt模板.pptx”,这是一个可以直接在PowerPoint软件中打开和编辑的演示文稿文件。用户可以根据自己的具体需要,在模板的基础上进行修改和补充,以制作出一个具有个性化特色的毕业设计答辩PPT。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
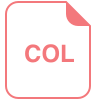
提升点阵式液晶显示屏效率技术
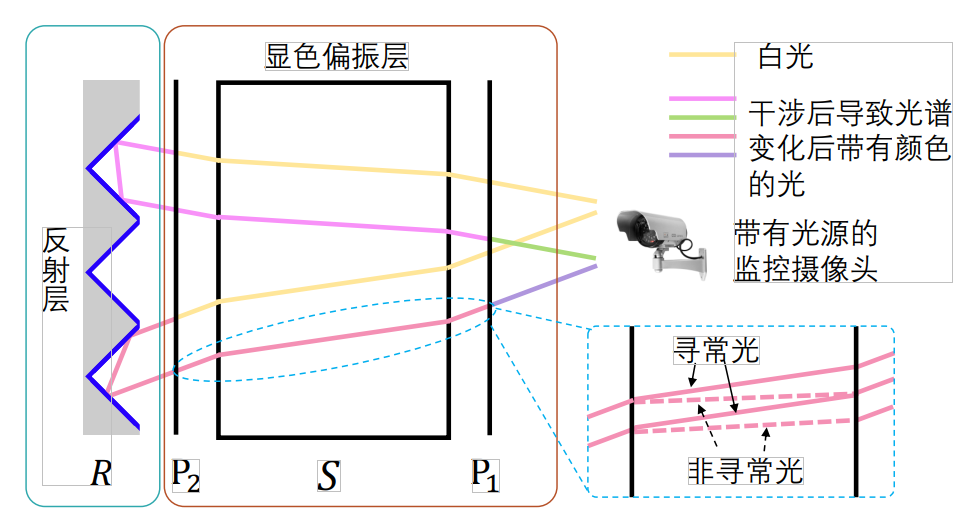
# 1. 点阵式液晶显示屏基础与效率挑战
在现代信息技术的浪潮中,点阵式液晶显示屏作为核心显示技术之一,已被广泛应用于从智能手机到工业控制等多个领域。本章节将介绍点阵式液晶显示屏的基础知识,并探讨其在提升显示效率过程中面临的挑战。
## 1.1 点阵式显

在SoC芯片的射频测试中,ATE设备通常如何执行系统级测试以保证芯片量产的质量和性能一致?
SoC芯片的射频测试是确保无线通信设备性能的关键环节。为了在量产阶段保证芯片的质量和性能一致性,ATE(Automatic Test Equipment)设备通常会执行一系列系统级测试。这些测试不仅关注芯片的电气参数,还包含电磁兼容性和射频信号的完整性检验。在ATE测试中,会根据芯片设计的规格要求,编写定制化的测试脚本,这些脚本能够模拟真实的无线通信环境,检验芯片的射频部分是否能够准确处理信号。系统级测试涉及对芯片基带算法的验证,确保其能够有效执行无线信号的调制解调。测试过程中,ATE设备会自动采集数据并分析结果,对于不符合标准的芯片,系统能够自动标记或剔除,从而提高测试效率和减少故障率。为了
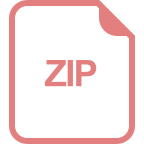
CodeSandbox实现ListView快速创建指南
资源摘要信息:"listview:用CodeSandbox创建"
知识点一:CodeSandbox介绍
CodeSandbox是一个在线代码编辑器,专门为网页应用和组件的快速开发而设计。它允许用户即时预览代码更改的效果,并支持多种前端开发技术栈,如React、Vue、Angular等。CodeSandbox的特点是易于使用,支持团队协作,以及能够直接在浏览器中编写代码,无需安装任何软件。因此,它非常适合初学者和快速原型开发。
知识点二:ListView组件
ListView是一种常用的用户界面组件,主要用于以列表形式展示一系列的信息项。在前端开发中,ListView经常用于展示从数据库或API获取的数据。其核心作用是提供清晰的、结构化的信息展示方式,以便用户可以方便地浏览和查找相关信息。
知识点三:用JavaScript创建ListView
在JavaScript中创建ListView通常涉及以下几个步骤:
1. 创建HTML的ul元素作为列表容器。
2. 使用JavaScript的DOM操作方法(如document.createElement, appendChild等)动态创建列表项(li元素)。
3. 将创建的列表项添加到ul容器中。
4. 通过CSS来设置列表和列表项的样式,使其符合设计要求。
5. (可选)为ListView添加交互功能,如点击事件处理,以实现更丰富的用户体验。
知识点四:在CodeSandbox中创建ListView
在CodeSandbox中创建ListView可以简化开发流程,因为它提供了一个在线环境来编写代码,并且支持实时预览。以下是使用CodeSandbox创建ListView的简要步骤:
1. 打开CodeSandbox官网,创建一个新的项目。
2. 在项目中创建或编辑HTML文件,添加用于展示ListView的ul元素。
3. 创建或编辑JavaScript文件,编写代码动态生成列表项,并将它们添加到ul容器中。
4. 使用CodeSandbox提供的实时预览功能,即时查看ListView的效果。
5. 若有需要,继续编辑或添加样式文件(通常是CSS),对ListView进行美化。
6. 利用CodeSandbox的版本控制功能,保存工作进度和团队协作。
知识点五:实践案例分析——listview-main
文件名"listview-main"暗示这可能是一个展示如何使用CodeSandbox创建基本ListView的项目。在这个项目中,开发者可能会包含以下内容:
1. 使用React框架创建ListView的示例代码,因为React是目前较为流行的前端库。
2. 展示如何将从API获取的数据渲染到ListView中,包括数据的获取、处理和展示。
3. 提供基本的样式设置,展示如何使用CSS来美化ListView。
4. 介绍如何在CodeSandbox中组织项目结构,例如如何分离组件、样式和脚本文件。
5. 包含一个简单的用户交互示例,例如点击列表项时弹出详细信息等。
总结来说,通过标题“listview:用CodeSandbox创建”,我们了解到本资源是一个关于如何利用CodeSandbox这个在线开发环境,来快速实现一个基于JavaScript的ListView组件的教程或示例项目。通过上述知识点的梳理,可以加深对如何创建ListView组件、CodeSandbox平台的使用方法以及如何在该平台中实现具体功能的理解。

"互动学习:行动中的多样性与论文攻读经历"
多样性她- 事实上SCI NCES你的时间表ECOLEDO C Tora SC和NCESPOUR l’Ingén学习互动,互动学习以行动为中心的强化学习学会互动,互动学习,以行动为中心的强化学习计算机科学博士论文于2021年9月28日在Villeneuve d'Asq公开支持马修·瑟林评审团主席法布里斯·勒菲弗尔阿维尼翁大学教授论文指导奥利维尔·皮耶昆谷歌研究教授:智囊团论文联合主任菲利普·普雷教授,大学。里尔/CRISTAL/因里亚报告员奥利维耶·西格德索邦大学报告员卢多维奇·德诺耶教授,Facebook /索邦大学审查员越南圣迈IMT Atlantic高级讲师邀请弗洛里安·斯特鲁布博士,Deepmind对于那些及时看到自己错误的人...3谢谢你首先,我要感谢我的两位博士生导师Olivier和Philippe。奥利维尔,"站在巨人的肩膀上"这句话对你来说完全有意义了。从科学上讲,你知道在这篇论文的(许多)错误中,你是我可以依
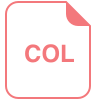
点阵式显示屏常见故障诊断方法
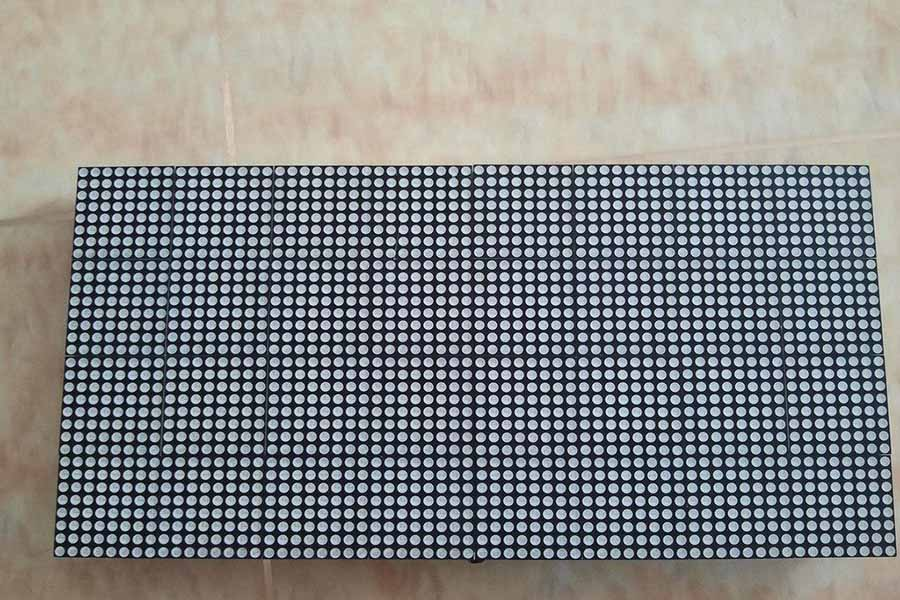
# 1. 点阵式显示屏的工作原理和组成
## 工作原理简介
点阵式显示屏的工作原理基于矩阵排列的像素点,每个像素点可以独立地被控制以显示不同的颜色和亮度,从而组合成复杂和精细的图像。其核心是通过驱动电路对各个LED或液晶单元进行单独控制,实现了图像的呈现。
## 显示屏的组成元素
组成点阵式显示屏的主要元素包括显示屏面板、驱动电路、控制单元和电源模块。面板包含了像素点矩阵,驱动电路则负责对像素点进行电

名词性从句包括哪些类别?它们各自有哪些引导词?请结合例句详细解释。
名词性从句分为四种:主语从句、宾语从句、表语从句和同位语从句。每种从句都有其特定的引导词,它们在句中承担不同的语法功能。要掌握名词性从句的运用,了解这些引导词的用法是关键。让我们深入探讨。
参考资源链接:[名词性从句解析:定义、种类与引导词](https://wenku.csdn.net/doc/bp0cjnmxco?spm=1055.2569.3001.10343)
首先,主语从句通常由whether, if, what, who, whose, how等引导词引导。它在句子中担任主语的角色,如例句'Whether he comes or not makes no differe