用QT C++实现输入底面半径和高计算并输出圆柱体的表面积和体积
时间: 2024-09-19 22:02:18 浏览: 42
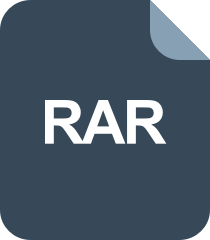
calculate_计算球、圆柱的表面积体积_
在Qt C++中,你可以使用QApplication、QInputDialog以及cmath库来实现这样的功能。首先,你需要创建一个窗口,并从用户那里获取输入的底面半径和高。然后,计算圆柱体的表面积和体积。下面是简单的步骤:
1. 导入所需的头文件:
```cpp
#include <QApplication>
#include <QInputDialog>
#include <cmath>
```
2. 创建主函数并初始化Qt应用程序:
```cpp
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
// 程序主体
QWidget window;
QVBoxLayout layout(&window);
QPushButton *calculateButton = new QPushButton("Calculate", &window);
QLineEdit *radiusInput = new QLineEdit(&window);
QLineEdit *heightInput = new QLineEdit(&window);
// 添加到布局中
layout.addWidget(radiusInput);
layout.addWidget(heightInput);
layout.addWidget(calculateButton);
window.setLayout(layout);
calculateButton->connect(calculateButton, &QPushButton::clicked,
this, &yourFunctionName); // 这里需要替换为实际的函数名
window.show();
return app.exec();
}
```
3. 定义计算表面积和体积的函数`yourFunctionName`:
```cpp
double calculateSurfaceAreaAndVolume(double radius, double height)
{
const double pi = M_PI; // 使用cmath库中的π值
// 计算表面积 (2πrh + 2πr²)
double surfaceArea = 2 * pi * radius * height + 2 * pi * radius * radius;
// 计算体积 (πr²h)
double volume = pi * pow(radius, 2) * height;
// 输出结果
qDebug() << "Table Surface Area: " << surfaceArea;
qDebug() << "Volume: " << volume;
return volume;
}
// 当按钮点击时调用这个函数
void yourFunctionName()
{
// 获取用户输入
double radius = qAtoi(QInputDialog::getText(nullptr, "Cylinder Calculator", "Enter the base radius:").toStdString());
double height = qAtoInt(QInputDialog::getText(nullptr, "Cylinder Calculator", "Enter the height:").toStdString());
if (!radius || !height)
return;
// 调用计算函数
double result = calculateSurfaceAreaAndVolume(radius, height);
}
```
当你运行程序,用户将能够输入圆柱体的半径和高,点击按钮后会计算并显示表面积和体积。
阅读全文
相关推荐
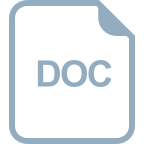
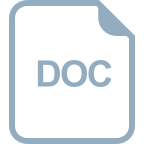


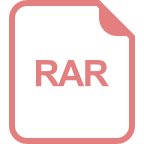
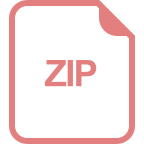
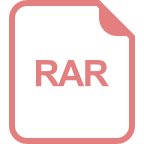
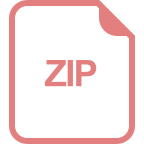
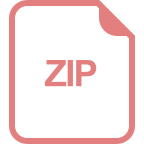
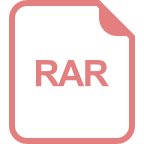
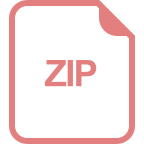
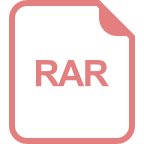
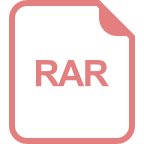
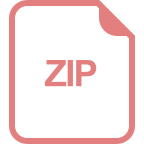
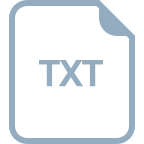

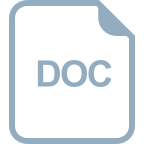