kotlin中自定义键盘
时间: 2023-12-22 07:33:37 浏览: 209
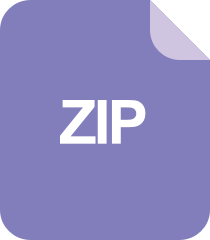
自定义键盘
在 Kotlin 中,你可以通过自定义键盘来实现特定的输入需求。下面是一个简单的例子,演示如何创建一个自定义键盘:
首先,创建一个自定义的键盘布局文件,例如 `custom_keyboard.xml`:
```xml
<Keyboard xmlns:android="http://schemas.android.com/apk/res/android"
android:keyWidth="33%p"
android:keyHeight="10%p">
<Row>
<Key android:keyLabel="A" />
<Key android:keyLabel="B" />
<Key android:keyLabel="C" />
</Row>
<Row>
<Key android:keyLabel="D" />
<Key android:keyLabel="E" />
<Key android:keyLabel="F" />
</Row>
<!-- 添加更多的行和按键 -->
</Keyboard>
```
然后,在你的 Activity 中,使用自定义键盘布局文件创建一个自定义键盘视图,并将其与 EditText 关联起来:
```kotlin
// 导入必要的类
import android.inputmethodservice.Keyboard
import android.inputmethodservice.KeyboardView
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
class MainActivity : AppCompatActivity() {
private lateinit var keyboardView: KeyboardView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val editText = findViewById<EditText>(R.id.editText)
// 创建自定义键盘
val customKeyboard = Keyboard(this, R.xml.custom_keyboard)
keyboardView = findViewById(R.id.keyboardView)
keyboardView.keyboard = customKeyboard
// 将键盘视图与 EditText 关联起来
keyboardView.setOnKeyboardActionListener(object : KeyboardView.OnKeyboardActionListener {
override fun onPress(primaryCode: Int) {}
override fun onRelease(primaryCode: Int) {}
override fun onKey(primaryCode: Int, keyCodes: IntArray?) {
val editable = editText.text
val start = editText.selectionStart
when (primaryCode) {
Keyboard.KEYCODE_DELETE -> {
if (editable.isNotEmpty() && start > 0) {
editable.delete(start - 1, start)
}
}
else -> {
editable.insert(start, primaryCode.toChar().toString())
}
}
}
override fun onText(text: CharSequence?) {}
override fun swipeLeft() {}
override fun swipeRight() {}
override fun swipeDown() {}
override fun swipeUp() {}
})
}
}
```
这是一个简单的示例,演示了如何在 Kotlin 中创建一个自定义键盘。你可以根据自己的需求进行修改和扩展。希望对你有所帮助!
阅读全文
相关推荐
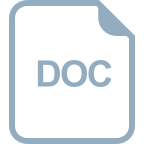
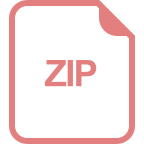
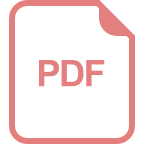
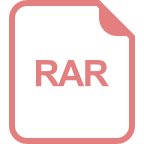
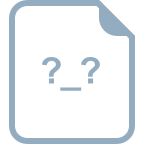

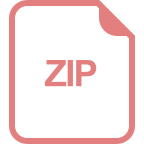
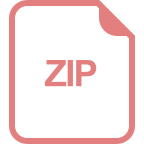
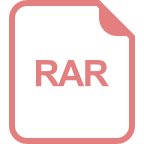
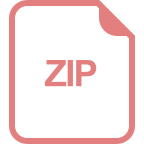
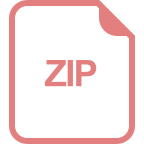
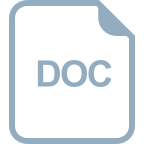
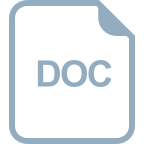
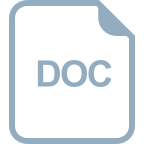
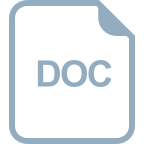
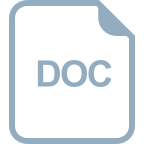
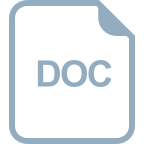