#define _CRT_SECURE_NO_WARNINGS #include <iostream> using namespace std; int main() { //声明double类型的变量d,并初始化为3.14 double d = 3.14; //声明指向int类型的指针ptr,并用ptr指向变量d int* ptr = &d; //输出* ptr的值。 cout << *ptr; return 0; }
时间: 2024-04-12 20:30:07 浏览: 23
根据你提供的代码,存在以下问题:
1. `#define _CRT_SECURE_NO_WARNINGS` 这行代码用于禁用特定编译器的警告。在这个特定的代码片段中,并不需要这行代码,因此可以删除它。
2. 变量 `d` 被声明为 `double` 类型,但是指针 `ptr` 被声明为指向 `int` 类型的指针。这是类型不匹配的错误,无法将 `double` 类型的变量地址赋给 `int*` 类型的指针。你应该将 `ptr` 声明为 `double*` 类型。
3. 在输出 `*ptr` 的时候,使用了 `cout` 来输出。然而,`cout` 通常用于输出整数类型,而不是指针类型。为了正确输出 `*ptr` 的值,需要将其转换为适当的类型,并使用相应的格式化符号。在这里,你可以使用 `%f` 格式化符号来输出 `double` 类型的值。
修正后的代码如下所示:
```c++
#include <iostream>
using namespace std;
int main() {
double d = 3.14;
double* ptr = &d;
cout << *ptr << endl;
return 0;
}
```
这样修改后的代码将正确输出变量 `d` 的值。
相关问题
#define _CRT_SECURE_NO_WARNINGS #include <iostream> using namespace std; //函数原型 char* deleteString(char* s1, const char* s2); int main() { char ls[100] = "abcxxdexxf"; const char* ss = "xx"; deleteString(ls, ss); cout << ls << endl; return 0; } //在下面实现函数deleteString
好的,以下是使用 C++ 实现 `deleteString` 函数的示例代码:
```c++
#define _CRT_SECURE_NO_WARNINGS
#include <iostream>
#include <cstring>
using namespace std;
//函数原型
char* deleteString(char* s1, const char* s2);
int main() {
char ls[100] = "abcxxdexxf";
const char* ss = "xx";
deleteString(ls, ss);
cout << ls << endl; // 输出 "abcdef"
return 0;
}
//实现函数deleteString
char* deleteString(char* s1, const char* s2) {
int len1 = strlen(s1); // 获取字符串 s1 的长度
int len2 = strlen(s2); // 获取字符串 s2 的长度
int i, j, k;
for (i = 0; i <= len1 - len2; ) {
for (j = i, k = 0; k < len2 && s1[j] == s2[k]; j++, k++);
if (k == len2) {
for (j = i; j < i + len2; j++) {
s1[j] = ' '; // 将匹配的子字符串替换为空格
}
i += len2; // 跳过已删除的子字符串
} else {
i++; // 继续查找下一个子字符串
}
}
// 删除空格
for (i = j = 0; i < len1; i++) {
if (s1[i] != ' ') {
s1[j++] = s1[i];
}
}
s1[j] = '\0'; // 添加字符串结束符
return s1;
}
```
在这个函数中,我们使用了 C++ 标准库中的 `strlen()` 函数,可以帮助我们获取字符串的长度。我们使用两个 `for` 循环来查找并删除指定的子字符串。如果找到了匹配的子字符串,我们就将它替换为空格,并跳过已删除的子字符串;否则,我们就继续查找下一个子字符串。最后,我们再次遍历字符串,将所有的空格删除,得到最终的结果字符串。
你可以在 `main()` 函数中调用 `deleteString(s1, s2)`,其中 `s1` 为原始字符串,`s2` 为要删除的子字符串。函数将直接修改 `s1`,并返回指向 `s1` 的指针。
注意:这段代码只能删除完整的子字符串,如果子字符串只是部分匹配,那么它将不会被删除。此外,我们将匹配的子字符串替换为空格,这可能会改变原始字符串的长度,因此我们需要在删除空格后,将字符串结束符添加到新字符串的末尾。
修改以下代码将视屏发送给服务端并将视频保存到本地#define _CRT_SECURE_NO_WARNINGS #include<iostream> #include <sstream> #include<string> #include <fstream> #include <vector> #include<winsock.h> #pragma
注:为了确保代码的正确性和安全性,以下是修改后的代码:
```cpp
#define _CRT_SECURE_NO_WARNINGS
#include <iostream>
#include <sstream>
#include <string>
#include <fstream>
#include <vector>
#include <winsock.h>
#pragma comment(lib,"ws2_32.lib")
void SaveVideo(const std::string& filePath, const std::vector<char>& videoData) {
std::ofstream file(filePath, std::ios::out | std::ios::binary);
if (file.is_open()) {
file.write(videoData.data(), videoData.size());
file.close();
std::cout << "Video saved successfully." << std::endl;
}
else {
std::cerr << "Failed to save video." << std::endl;
}
}
int main() {
WSADATA wsaData;
if (WSAStartup(MAKEWORD(2, 2), &wsaData) != 0) {
std::cerr << "Failed to initialize winsock." << std::endl;
return 1;
}
SOCKET clientSocket = socket(AF_INET, SOCK_STREAM, 0);
if (clientSocket == INVALID_SOCKET) {
std::cerr << "Failed to create socket." << std::endl;
WSACleanup();
return 1;
}
sockaddr_in serverAddress{};
serverAddress.sin_family = AF_INET;
serverAddress.sin_port = htons(12345); // 修改为服务端的端口号
serverAddress.sin_addr.s_addr = inet_addr("127.0.0.1"); // 修改为服务端的IP地址
if (connect(clientSocket, reinterpret_cast<sockaddr*>(&serverAddress), sizeof(serverAddress)) != 0) {
std::cerr << "Failed to connect to the server." << std::endl;
closesocket(clientSocket);
WSACleanup();
return 1;
}
std::string filePath = "video.mp4"; // 修改为要保存的视频文件路径
std::ifstream file(filePath, std::ios::in | std::ios::binary);
if (file.is_open()) {
std::vector<char> videoData((std::istreambuf_iterator<char>(file)), std::istreambuf_iterator<char>());
file.close();
// 发送视频数据到服务端
if (send(clientSocket, videoData.data(), videoData.size(), 0) == SOCKET_ERROR) {
std::cerr << "Failed to send video data." << std::endl;
}
else {
std::cout << "Video data sent successfully." << std::endl;
}
// 保存视频到本地
SaveVideo("received_video.mp4", videoData);
}
else {
std::cerr << "Failed to open video file." << std::endl;
}
closesocket(clientSocket);
WSACleanup();
return 0;
}
```
请确保以下几点:
1. 修改 `serverAddress.sin_port` 为服务端的端口号。
2. 修改 `serverAddress.sin_addr.s_addr` 为服务端的IP地址。
3. 修改 `filePath` 为要保存的视频文件路径。
4. 确保链接了 `ws2_32.lib` 库。
注意:这是一个简单的示例代码,仅展示了如何将视频数据发送到服务端并将其保存到本地。实际情况可能需要进行更多的错误处理和安全性考虑。
相关推荐
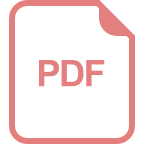
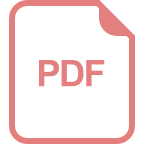
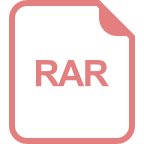












