2.有顺序表A和B,其元素均按从小到大的升序排列,用C语言编写一个算法,将它们合并成一个顺序表C,要求C的元素也按从小到大的升序排列。
时间: 2024-09-20 10:03:16 浏览: 58
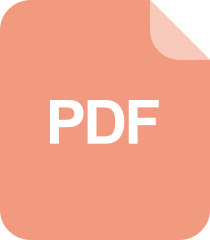
C语言的排序算法.pdf
要将两个已排序的顺序表A和B合并成一个新的有序顺序表C,你可以使用双指针法。以下是使用C语言的一个简单示例:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
Node* mergeSortedLists(Node* list1, Node* list2) {
// 创建新节点作为结果列表的头结点
Node* head = (list1 == NULL) ? list2 : (list2 == NULL) ? list1 : (list1->data <= list2->data) ? list1 : list2;
// 当其中一个列表为空时,将另一个列表剩余部分添加到结果中
if (head != list1) {
list1 = list1->next;
}
if (head != list2) {
list2 = list2->next;
}
// 使用临时变量存储当前遍历的节点
Node* current = head;
while (list1 && list2) {
if (list1->data <= list2->data) {
current->next = list1;
list1 = list1->next;
} else {
current->next = list2;
list2 = list2->next;
}
current = current->next;
}
// 如果其中一个列表还有剩余元素,将其直接添加到结果列表末尾
if (list1) {
current->next = list1;
} else {
current->next = list2;
}
return head;
}
int main() {
// 假设已经创建了顺序表A和B的头结点,并设置了它们的值
Node* A = ...; // 顺序表A的头结点
Node* B = ...; // 顺序表B的头结点
// 合并两个列表
Node* C = mergeSortedLists(A, B);
// 打印合并后的顺序表C
while (C) {
printf("%d ", C->data);
C = C->next;
}
return 0;
}
```
阅读全文
相关推荐
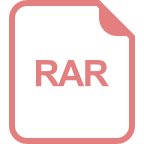













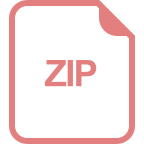