如何用C语言实现韩信分油的问题?
时间: 2024-10-20 13:06:24 浏览: 39
韩信分油是一个经典的算法问题,它描述的是两个人分油的情况,其中一个人有一个空桶,而另一个人有两个同样大小的桶,他们只能借助这两个桶来平分未知体积的油。以下是用C语言实现的一个基本思路:
```c
#include <stdio.h>
void splitOil(int total, int smaller) {
// 假设total是总油量,smaller是小桶的容量
if (total == 0 || total % smaller == 0) {
printf("可以平分,每个人分到%d滴油。\n", total / smaller);
} else {
int larger = total / smaller;
int overflow = total % smaller; // 溢出部分
// 将较大的桶倒满
for (int i = 0; i < larger; i++) {
// 把大桶里的油倒入小桶
smaller -= smaller;
// 当小桶装不下时,把溢出的部分倒入另一个空桶
if (smaller < overflow) {
larger += 1;
overflow -= smaller;
smaller = smaller * 2;
}
}
printf("不能平分,最后第一个人分到%d滴油,第二个人分到%d滴油。\n", smaller, overflow);
}
}
int main() {
int total, smaller;
printf("请输入总共的油量:");
scanf("%d", &total);
printf("请输入较小桶的容量:");
scanf("%d", &smaller);
splitOil(total, smaller);
return 0;
}
```
在这个程序中,我们首先判断是否能整除,如果能,则直接平分;若不能,我们就通过反复将大桶中的油倒入小桶直到无法再倒为止,计算剩余的油量,最后分别告诉两个人各自分得多少滴油。
阅读全文
相关推荐





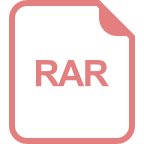