分别用小批量梯度下降和随机梯度下降求解C:\\Users\\孙立华\\Downloads\\concrete.csv混凝土数据集(取前8列分析)中混凝土强度与各个因素之间的系数theta,写出符合上述要求的完整代码
时间: 2024-04-21 14:21:13 浏览: 93
使用小批量梯度下降和随机梯度下降求解混凝土数据集中混凝土强度与各个因素之间的系数theta的代码如下:
```python
import numpy as np
import pandas as pd
# 读取数据集
data = pd.read_csv('C:\\Users\\孙立华\\Downloads\\concrete.csv')
X = data.iloc[:, :8].values
y = data.iloc[:, 8].values
# 特征归一化
X = (X - np.mean(X, axis=0)) / np.std(X, axis=0)
# 添加偏置项
X = np.c_[np.ones(X.shape), X]
# 定义损失函数
def compute_cost(X, y, theta):
m = len(y)
predictions = X.dot(theta)
cost = (1/(2*m)) * np.sum((predictions-y)**2)
return cost
# 定义小批量梯度下降函数
def mini_batch_gradient_descent(X, y, theta, learning_rate=0.01, batch_size=32, num_iterations=1000):
m = len(y)
costs = []
for i in range(num_iterations):
# 随机选择小批量样本
indices = np.random.randint(0, m, batch_size)
X_batch = X[indices]
y_batch = y[indices]
# 计算梯度
predictions = X_batch.dot(theta)
errors = predictions - y_batch
gradient = (1/batch_size) * X_batch.T.dot(errors)
# 更新参数
theta = theta - learning_rate * gradient
# 计算损失函数
cost = compute_cost(X, y, theta)
costs.append(cost)
return theta, costs
# 定义随机梯度下降函数
def stochastic_gradient_descent(X, y, theta, learning_rate=0.01, num_iterations=1000):
m = len(y)
costs = []
for i in range(num_iterations):
# 随机选择一个样本
index = np.random.randint(0, m)
X_sample = X[index]
y_sample = y[index]
# 计算梯度
prediction = X_sample.dot(theta)
error = prediction - y_sample
gradient = X_sample.T.dot(error)
# 更新参数
theta = theta - learning_rate * gradient
# 计算损失函数
cost = compute_cost(X, y, theta)
costs.append(cost)
return theta, costs
# 初始化参数
theta_init = np.zeros(X.shape)
# 使用小批量梯度下降求解theta
theta_mini_batch, costs_mini_batch = mini_batch_gradient_descent(X, y, theta_init)
# 使用随机梯度下降求解theta
theta_stochastic, costs_stochastic = stochastic_gradient_descent(X, y, theta_init)
# 打印结果
print("使用小批量梯度下降求解得到的theta:", theta_mini_batch)
print("使用随机梯度下降求解得到的theta:", theta_stochastic)
# 绘制损失函数曲线
import matplotlib.pyplot as plt
plt.plot(range(len(costs_mini_batch)), costs_mini_batch, label='Mini-Batch Gradient Descent')
plt.plot(range(len(costs_stochastic)), costs_stochastic, label='Stochastic Gradient Descent')
plt.xlabel('Iterations')
plt.ylabel('Cost')
plt.legend()
plt.show()
```
阅读全文
相关推荐
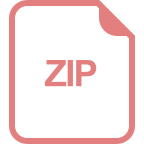
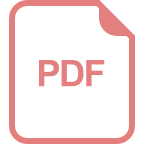
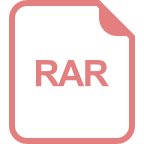
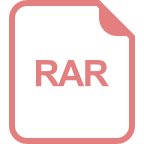
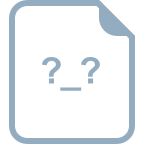
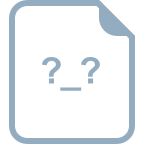
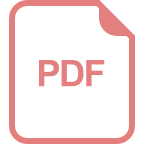
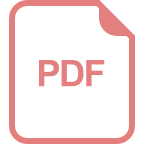
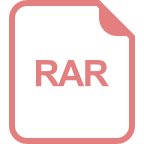
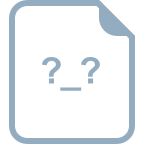
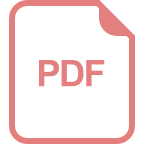
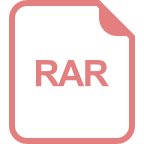
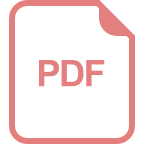
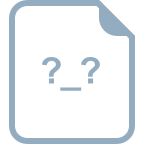
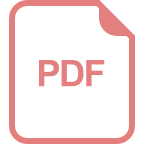
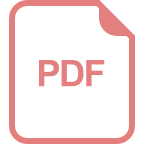