用c语言指针实现统计字符串中某一子字符串出现的次数,不用函数调用
时间: 2024-12-10 15:51:14 浏览: 44
在C语言中,你可以通过遍历整个字符串,并用指针来查找目标子串,同时记录子串出现的次数。下面是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
int count_substring(char *str, char *substr) {
int i = 0, j = 0, count = 0;
while (i < strlen(str)) {
// 如果当前子串匹配,计数加一并移动到下一个位置
if (str[i] == substr[j]) {
i++;
j++;
if (j == strlen(substr)) { // 子串完全匹配
count++;
i = i - strlen(substr); // 移动主指针回到子串结束的位置
j = 0; // 重置子串指针
}
} else {
i = i - j + 1; // 没有匹配,回退到上次匹配后的位置
j = 0; // 重置子串指针
}
}
return count;
}
int main() {
char str[] = "Hello, World! Hello again!";
char substr[] = "Hello";
int occurrences = count_substring(str, substr);
printf("Substring '%s' appears %d times in the given string.\n", substr, occurrences);
return 0;
}
```
在这个例子中,`count_substring` 函数接收两个字符串作为输入,主字符串 `str` 和需要查找的子字符串 `substr`。它使用两个指针 `i` 和 `j` 分别跟踪主字符串和子字符串的当前位置。如果找到子串,就递增计数;否则,回退到上一次匹配的位置继续搜索。
阅读全文
相关推荐
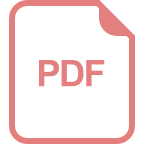
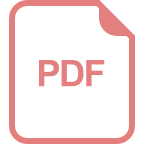
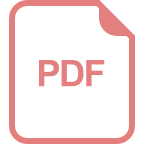
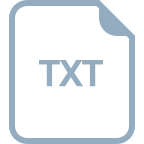
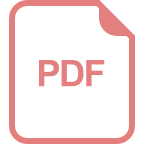
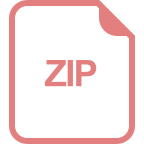
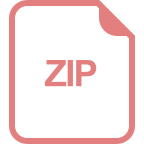
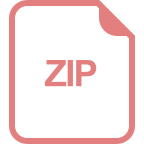
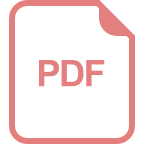
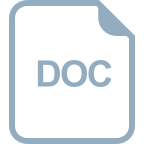
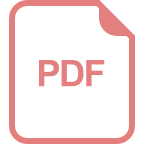
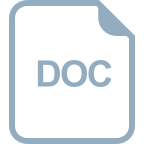
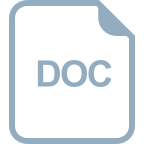
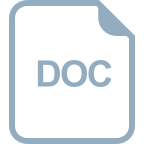
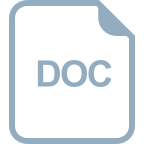
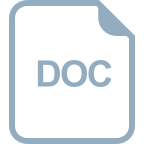
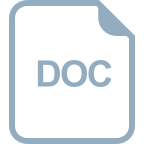