string folderPath = "C:\\path\\to\\folder"; SendEmailsInFolder(outlookApp, folderPath);
时间: 2023-08-12 08:03:40 浏览: 33
根据你提供的代码片段,似乎是想发送指定文件夹中的邮件及其附件。以下是一个使用 C# 开发的示例程序,实现了这个功能:
```csharp
using System;
using System.IO;
using Outlook = Microsoft.Office.Interop.Outlook;
namespace OutlookMailApp
{
class Program
{
static void Main(string[] args)
{
// 连接 Outlook
Outlook.Application outlookApp = new Outlook.Application();
// 发送指定文件夹中的邮件及其附件
string folderPath = "C:\\path\\to\\folder";
SendEmailsInFolder(outlookApp, folderPath);
// 断开与 Outlook 的连接
System.Runtime.InteropServices.Marshal.ReleaseComObject(outlookApp);
}
static void SendEmailsInFolder(Outlook.Application outlookApp, string folderPath)
{
string[] files = Directory.GetFiles(folderPath);
foreach (string file in files)
{
// 创建新邮件项
Outlook.MailItem mailItem = (Outlook.MailItem)outlookApp.CreateItem(Outlook.OlItemType.olMailItem);
mailItem.Subject = "Email with Attachment";
mailItem.Body = "Please find the attached file.";
// 添加附件
mailItem.Attachments.Add(file);
// 设置收件人
Outlook.Recipients recipients = mailItem.Recipients;
Outlook.Recipient recipient = recipients.Add("recipient@example.com");
recipient.Resolve();
// 发送邮件
mailItem.Send();
// 释放资源
System.Runtime.InteropServices.Marshal.ReleaseComObject(mailItem);
System.Runtime.InteropServices.Marshal.ReleaseComObject(recipient);
System.Runtime.InteropServices.Marshal.ReleaseComObject(recipients);
}
}
}
}
```
在上述代码中,我们使用了 Microsoft.Office.Interop.Outlook 命名空间下的 Outlook.Application、Outlook.MailItem、Outlook.Recipients 和 Outlook.Recipient 类来连接 Outlook、创建新邮件项、添加附件以及发送邮件。请确保在运行程序之前已安装并配置了 Outlook,并将目标文件夹的路径和收件人的邮箱地址替换为你实际使用的值。
相关推荐
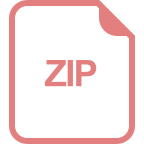
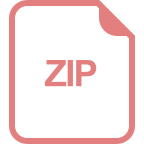
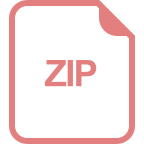














