从文本中读取出指定学号的学生信息并显示文本文件存放格式是每一行都有一个学生信息最后一行没有换行符
时间: 2024-01-01 15:23:38 浏览: 48
你可以使用以下代码从文本中读取出指定学号的学生信息并显示:
```python
def read_student_info(student_id):
with open('student.txt', 'r') as file:
for line in file:
info = line.strip().split('\t')
if info[2] == student_id:
return info
return None
student_id = '123456' # 指定学号
student_info = read_student_info(student_id)
if student_info:
print("学生信息:")
print("姓名:", student_info[0])
print("年龄:", student_info[1])
print("学号:", student_info[2])
print("平均分:", student_info[3])
else:
print("未找到该学号的学生信息")
```
请注意,上述代码假设学生信息文件名为`student.txt`,每行都有一个学生信息,最后一行没有换行符,并且学生信息以制表符`\t`分隔。
相关问题
实现从文本中读取出指定学号的学生信息并显示,文本文件存放格式是每一行对应一个学生信息,最后一行没有换符
你可以使用以下代码来实现从文本中读取出指定学号的学生信息并显示:
```python
def get_student_info(student_id):
with open('student.txt', 'r') as file:
for line in file:
info = line.strip().split('\t')
if info[2] == student_id:
return info
return None
student_id = '123456' # 指定学号
student_info = get_student_info(student_id)
if student_info:
print("学生信息:")
print("姓名:", student_info[0])
print("年龄:", student_info[1])
print("学号:", student_info[2])
print("平均分:", student_info[3])
else:
print("未找到该学号对应的学生信息")
```
实现从文本中读取出指定学号的学生信息并显示,文本文件存放格式是每一行对应一个学生信息,最后一行没有换行符。
以下是实现从文本中读取出指定学号的学生信息并显示的Python代码:
```python
def get_student_info(student_id):
with open('student.txt', 'r') as file:
for line in file:
info = line.strip().split(',')
if info[0] == student_id:
return info
return None
student_id = input("请输入学号:")
student_info = get_student_info(student_id)
if student_info:
print("学生信息:", student_info)
else:
print("未找到该学生信息")
```
请注意,上述代码假设学生信息文件名为`student.txt`,每一行的格式为`学号,姓名,年龄,性别`。代码通过逐行读取文件内容,将每一行的信息按逗号分隔,并与输入的学号进行比较,如果找到匹配的学号,则返回该学生的信息。如果未找到匹配的学号,则返回None。
相关推荐
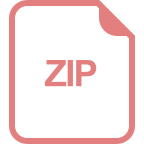
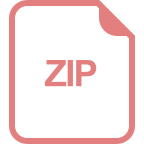
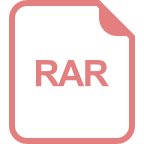






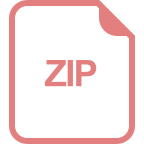
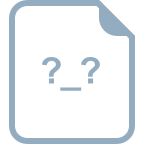
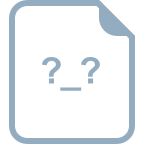
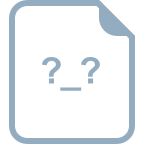
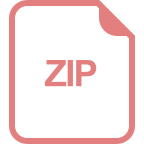
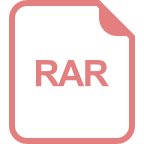
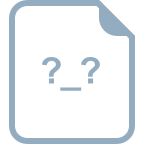
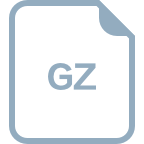