图书管理系统代码java
时间: 2024-05-28 14:07:29 浏览: 100
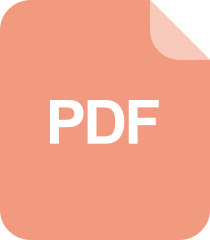
图书管理系统java代码.pdf
图书管理系统是一个常见的应用程序,主要用于管理图书馆、学校和企业等机构的图书信息。它可以帮助管理员快速准确地查询、添加、修改和删除图书信息,方便读者借阅图书。
Java是一种广泛应用于开发Web应用、桌面应用和移动应用的编程语言。以下是一个简单的图书管理系统的Java代码示例:
```
import java.util.ArrayList;
import java.util.Scanner;
public class BookManagementSystem {
private static ArrayList<Book> bookList = new ArrayList<Book>();
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int choice;
do {
System.out.println("1. Add Book");
System.out.println("2. Remove Book");
System.out.println("3. Search Book");
System.out.println("4. Display All Books");
System.out.println("5. Exit");
System.out.print("Enter your choice: ");
choice = input.nextInt();
switch (choice) {
case 1:
addBook();
break;
case 2:
removeBook();
break;
case 3:
searchBook();
break;
case 4:
displayAllBooks();
break;
case 5:
System.out.println("Exiting program...");
break;
default:
System.out.println("Invalid choice. Try again.");
}
} while (choice != 5);
}
private static void addBook() {
Scanner input = new Scanner(System.in);
System.out.print("Enter book title: ");
String title = input.nextLine();
System.out.print("Enter book author: ");
String author = input.nextLine();
System.out.print("Enter book ISBN: ");
String isbn = input.nextLine();
Book book = new Book(title, author, isbn);
bookList.add(book);
System.out.println("Book added successfully.");
}
private static void removeBook() {
Scanner input = new Scanner(System.in);
System.out.print("Enter book ISBN: ");
String isbn = input.nextLine();
for (int i = 0; i < bookList.size(); i++) {
Book book = bookList.get(i);
if (book.getIsbn().equals(isbn)) {
bookList.remove(i);
System.out.println("Book removed successfully.");
return;
}
}
System.out.println("Book not found.");
}
private static void searchBook() {
Scanner input = new Scanner(System.in);
System.out.print("Enter book title or author: ");
String keyword = input.nextLine();
for (int i = 0; i < bookList.size(); i++) {
Book book = bookList.get(i);
if (book.getTitle().contains(keyword) || book.getAuthor().contains(keyword)) {
System.out.println(book);
}
}
}
private static void displayAllBooks() {
for (int i = 0; i < bookList.size(); i++) {
System.out.println(bookList.get(i));
}
}
}
class Book {
private String title;
private String author;
private String isbn;
public Book(String title, String author, String isbn) {
this.title = title;
this.author = author;
this.isbn = isbn;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String getIsbn() {
return isbn;
}
public String toString() {
return "Title: " + title + ", Author: " + author + ", ISBN: " + isbn;
}
}
```
这段代码实现了基本的添加、删除、查询和显示所有图书的功能。您可以根据您自己的需求进行修改和扩展。如果您对这段代码有任何问题或需要更多的帮助,请随时问我。
阅读全文
相关推荐
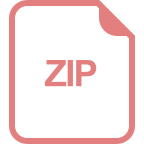
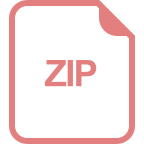
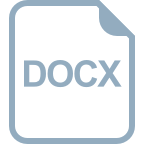
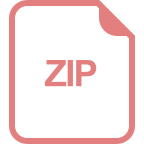
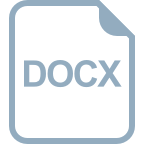
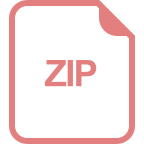
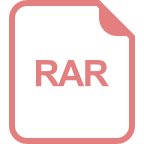
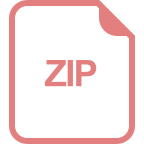