为我生成基于springboot的图书管理系统
时间: 2024-12-19 20:27:00 浏览: 3
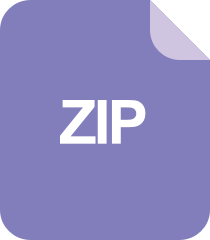
图书-图书系统-图书系统源码-图书管理系统-图书管理系统java代码-基于Web的图书系统设计与实现-图书系统设计与实现-代码
构建一个基于Spring Boot的图书管理系统通常会包括以下几个主要步骤:
1. **初始化项目**:使用Spring Initializr或其他工具创建一个新的Spring Boot项目,选择Web、JPA(用于数据访问)、Thymeleaf(HTML模板引擎)等依赖。
2. **实体类**(Book.java):
```java
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
private String author;
// getters and setters...
}
```
3. **数据访问层**(BookRepository.java):
```java
import org.springframework.data.jpa.repository.JpaRepository;
public interface BookRepository extends JpaRepository<Book, Long> {}
```
4. **服务层**(BookService.java):
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class BookService {
@Autowired
private BookRepository bookRepository;
public List<Book> getAllBooks() {
return bookRepository.findAll();
}
// 添加其他CRUD操作...
}
```
5. **控制器层**(BookController.java):
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class BookController {
@Autowired
private BookService bookService;
@GetMapping("/books")
public List<Book> getAllBooks() {
return bookService.getAllBooks();
}
}
```
6. **视图层**(views目录下的templates/book/index.html):
使用Thymeleaf展示图书列表。
7. **配置文件**(application.properties):配置数据库连接信息。
为了更完整地搭建系统,你还需要处理用户认证、分页、搜索等功能。此外,确保遵循良好的设计原则,如单一职责原则、开闭原则等。
阅读全文
相关推荐
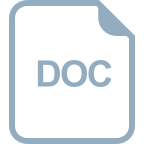
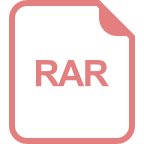
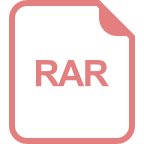
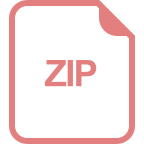
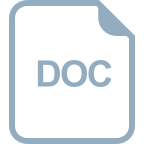
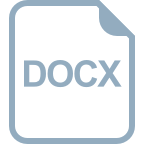
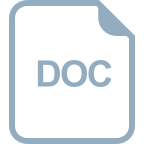
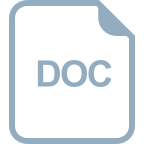
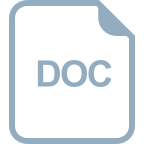
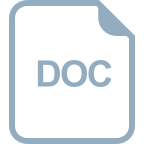
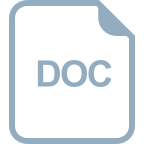




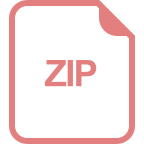
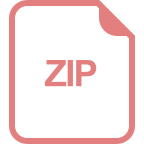