超市进销存系统代码设计
时间: 2023-08-26 18:05:45 浏览: 140
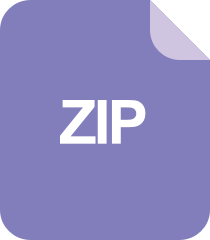
vb超市进销存管理系统设计(源代码+可执行文件+论文+开题报稿+外文翻译+答辩ppt).zip
设计一个超市进销存系统的代码需要考虑多个方面,包括商品管理、库存管理、销售管理等。以下是一个简单的代码设计示例,涵盖了基本功能:
```python
class Product:
def __init__(self, id, name, price, quantity):
self.id = id
self.name = name
self.price = price
self.quantity = quantity
class Inventory:
def __init__(self):
self.products = []
def add_product(self, product):
self.products.append(product)
def remove_product(self, product_id):
for product in self.products:
if product.id == product_id:
self.products.remove(product)
break
def update_product_quantity(self, product_id, quantity):
for product in self.products:
if product.id == product_id:
product.quantity = quantity
break
def get_product_quantity(self, product_id):
for product in self.products:
if product.id == product_id:
return product.quantity
return 0
class Sales:
def __init__(self):
self.sales = []
def add_sale(self, product_id, quantity):
self.sales.append({"product_id": product_id, "quantity": quantity})
def remove_sale(self, product_id):
for sale in self.sales:
if sale["product_id"] == product_id:
self.sales.remove(sale)
break
def get_total_sales(self):
total_sales = 0
for sale in self.sales:
total_sales += sale["quantity"]
return total_sales
# 示例用法
inventory = Inventory()
# 添加商品
product1 = Product(1, "Apple", 2.5, 10)
product2 = Product(2, "Banana", 1.5, 15)
inventory.add_product(product1)
inventory.add_product(product2)
# 更新商品库存
inventory.update_product_quantity(1, 5)
# 获取商品库存
print(inventory.get_product_quantity(1)) # 输出: 5
# 添加销售记录
sales = Sales()
sales.add_sale(1, 3)
sales.add_sale(2, 4)
# 获取总销售量
print(sales.get_total_sales()) # 输出: 7
```
上述代码示例中,`Product` 类用于表示商品,包含 id、名称、价格和数量等属性。`Inventory` 类用于管理商品库存,包含添加商品、移除商品、更新商品数量和获取商品数量等方法。`Sales` 类用于管理销售记录,包含添加销售记录、删除销售记录和获取总销售量等方法。
这只是一个简单的示例,实际的超市进销存系统可能需要更多的功能和复杂性考虑,比如商品分类、供应商管理、销售统计分析等。具体的代码设计还需根据你的需求和规模进行调整。
阅读全文
相关推荐
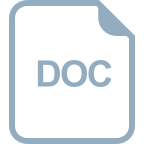
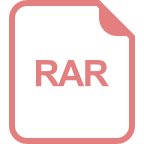
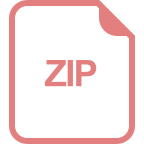
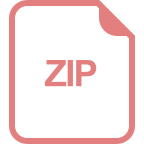
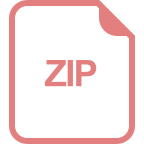
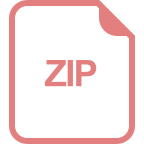
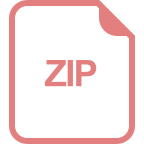
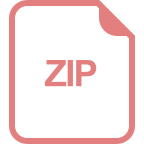

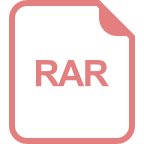
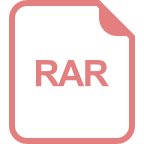
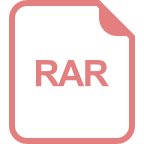
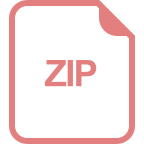
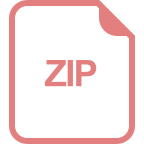
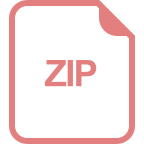