uniapp中点击按钮显示图片
时间: 2024-05-31 11:05:52 浏览: 11
在uniapp中,可以通过以下步骤实现点击按钮显示图片:
1. 在template中创建一个按钮元素,绑定一个点击事件:
```
<template>
<view>
<button @tap="showImage">显示图片</button>
<image v-if="isShowImage" src="/static/image.png"></image>
</view>
</template>
```
2. 在script中添加showImage方法,设置isShowImage为true,从而显示图片:
```
<script>
export default {
data() {
return {
isShowImage: false
}
},
methods: {
showImage() {
this.isShowImage = true
}
}
}
</script>
```
这样点击按钮后,就会显示一张名为image.png的图片。
相关问题
vue3.2 使用uniapp onShareAppMessage 添加点击按钮
在Vue3.2中,使用uniapp的onShareAppMessage方法添加自定义分享按钮的方法与Vue3中基本相同,只是添加自定义按钮的方式略有不同。
首先,在组件中添加onShareAppMessage方法,该方法会返回一个对象,用于配置分享信息。例如:
```
export default {
methods: {
onShareAppMessage() {
return {
title: '分享标题',
path: '/pages/index/index',
imageUrl: 'https://example.com/share.jpg',
success: function(res) {
console.log('分享成功', res)
}
}
}
}
}
```
其中,title为分享标题,path为分享路径,imageUrl为分享图片的链接,success为分享成功后的回调函数。
接着,在模板中添加一个自定义按钮,并绑定一个点击事件:
```
<template>
<view>
<button @tap="onCustomShare">自定义分享</button>
</view>
</template>
```
最后,在onCustomShare方法中调用uniapp提供的showShareMenu方法,打开分享弹窗,并且可以在弹窗中添加自定义按钮。例如:
```
export default {
methods: {
onCustomShare() {
uni.showShareMenu({
withShareTicket: true,
menus: ['shareAppMessage', 'shareTimeline'],
success: function() {
uni.onShareAppMessage(function() {
return {
title: '分享标题',
path: '/pages/index/index',
imageUrl: 'https://example.com/share.jpg',
success: function(res) {
console.log('分享成功', res)
}
}
})
uni.onShareTimeline(function() {
console.log('分享到朋友圈')
})
uni.showShareItems({
itemList: [
{
id: 'customShareButton',
iconPath: 'https://example.com/share-button.jpg',
title: '自定义按钮'
}
],
success: function(res) {
console.log('自定义分享按钮点击', res)
}
})
}
})
}
}
}
```
其中,withShareTicket表示是否开启分享到群的功能,menus表示分享弹窗中显示的按钮,uni.onShareAppMessage和uni.onShareTimeline分别用于配置分享到好友和分享到朋友圈的信息,uni.showShareItems用于添加自定义分享按钮。
总结起来,使用uniapp的onShareAppMessage方法可以方便地在Vue3.2中添加分享功能,并且还可以自定义分享弹窗中的按钮。
vue3 使用uniapp onShareAppMessage 添加点击按钮
在Vue3中,可以使用uniapp提供的onShareAppMessage方法来添加分享功能,并且可以在分享弹窗中添加自定义按钮。
首先,在组件中添加onShareAppMessage方法,该方法会返回一个对象,用于配置分享信息。例如:
```
export default {
methods: {
onShareAppMessage() {
return {
title: '分享标题',
path: '/pages/index/index',
imageUrl: 'https://example.com/share.jpg',
success: function(res) {
console.log('分享成功', res)
}
}
}
}
}
```
其中,title为分享标题,path为分享路径,imageUrl为分享图片的链接,success为分享成功后的回调函数。
接着,在模板中添加一个自定义按钮,并绑定一个点击事件:
```
<template>
<view>
<button @tap="onCustomShare">自定义分享</button>
</view>
</template>
```
最后,在onCustomShare方法中调用uniapp提供的showShareMenu方法,打开分享弹窗,并且可以在弹窗中添加自定义按钮。例如:
```
export default {
methods: {
onCustomShare() {
uni.showShareMenu({
withShareTicket: true,
menus: ['shareAppMessage', 'shareTimeline'],
success: function() {
uni.onShareAppMessage(function() {
return {
title: '分享标题',
path: '/pages/index/index',
imageUrl: 'https://example.com/share.jpg',
success: function(res) {
console.log('分享成功', res)
}
}
})
uni.onShareTimeline(function() {
console.log('分享到朋友圈')
})
uni.addShareButton({
title: '自定义按钮',
imageUrl: 'https://example.com/share-button.jpg',
success: function(res) {
console.log('自定义分享按钮点击', res)
}
})
}
})
}
}
}
```
其中,withShareTicket表示是否开启分享到群的功能,menus表示分享弹窗中显示的按钮,uni.onShareAppMessage和uni.onShareTimeline分别用于配置分享到好友和分享到朋友圈的信息,uni.addShareButton用于添加自定义分享按钮。
总结起来,使用uniapp的onShareAppMessage方法可以方便地在Vue3中添加分享功能,并且还可以自定义分享弹窗中的按钮。
相关推荐
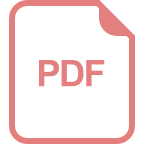
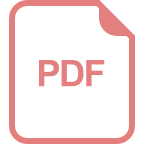












