Python场景描述: 1.用户订单处理:用户购买了两件商品A(单价100元)和一件商品B(单价200元),需要计算订单总价,并判 断用户是否享有满300元减50元的优惠。 2.库存更新:商品A和商品B的初始库存
时间: 2024-09-21 08:05:16 浏览: 31
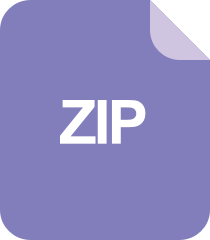
基于python实现的京东预约商品自动下单源码.zip

在Python中,我们可以模拟这样的场景:
1. **用户订单处理**:
```python
class Product:
def __init__(self, name, price):
self.name = name
self.price = price
class Order:
def __init__(self):
self.items = []
def add_item(self, product, quantity):
item = {"product": product, "quantity": quantity}
self.items.append(item)
def calculate_total(self):
total = sum([item["product"].price * item["quantity"] for item in self.items])
return total
def check_discount(self, threshold=300):
if self.calculate_total() >= threshold:
discount = 50
return total - discount
else:
return total
# 使用示例
product_a = Product("商品A", 100)
product_b = Product("商品B", 200)
order = Order()
order.add_item(product_a, 2) # 购买两件A
order.add_item(product_b, 1) # 购买一件B
total_with_discount = order.check_discount()
```
2. **库存更新**:
```python
class Inventory:
def __init__(self):
self.products = {
"商品A": 100,
"商品B": 100, # 初始化库存量假设都为100件
}
def update_stock(self, product, quantity, action="add"):
if action == "add":
self.products[product] += quantity
elif action == "subtract":
if self.products[product] >= quantity:
self.products[product] -= quantity
else:
print(f"库存不足,无法减少{product}到{quantity}件")
# 示例
inventory = Inventory()
inventory.update_stock("商品A", 2) # 更新商品A库存
```
阅读全文
相关推荐
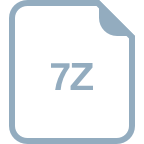
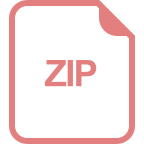

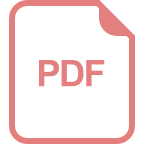
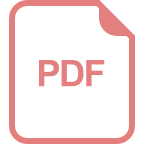
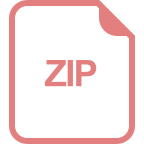
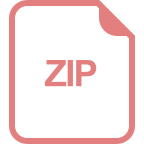
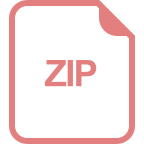
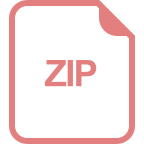
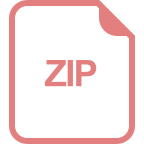
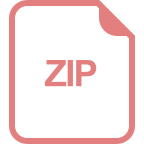
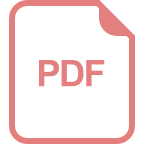
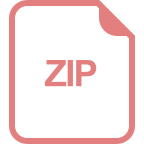
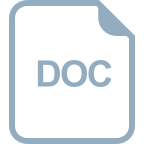
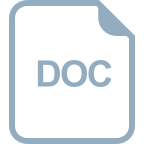
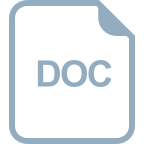
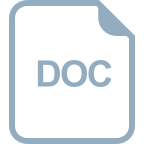