java eclipse 实训项目代码
时间: 2024-12-26 20:15:46 浏览: 7
### Java Eclipse 实训项目代码示例
#### 创建并配置Eclipse工程
为了在Eclipse中启动Java实训项目,需先安装好JDK以及Eclipse IDE。打开Eclipse之后,通过`File -> New -> Project...`来新建一个Java Project。
#### 编写复数类作为实例
下面展示了一个简单的复数(Complex Number)处理类的实现方式:
```java
public class Complex {
private double real;
private double imaginary;
public Complex(double r, double i){
this.real = r;
this.imaginary = i;
}
// 加法操作方法
public Complex add(Complex other){
return new Complex(this.real + other.real,
this.imaginary + other.imaginary);
}
// 减法操作方法
public Complex subtract(Complex other){
return new Complex(this.real - other.real,
this.imaginary - other.imaginary);
}
@Override
public String toString(){
return "(" + this.real + " + " + this.imaginary +"i)";
}
}
```
此段代码定义了两个基本运算——加法和减法,并重写了toString()以便于打印输出[^2]。
#### 测试复数类的功能
可以创建另一个名为TestComplex的测试类来进行验证:
```java
public class TestComplex {
public static void main(String[] args) {
Complex c1 = new Complex(3.0, 4.0); // 构造函数初始化
Complex c2 = new Complex(-1.0, 2.0);
System.out.println("C1: "+c1.toString());
System.out.println("C2: "+c2.toString());
Complex sum = c1.add(c2);
System.out.println("Sum of C1 and C2 is :"+sum.toString());
Complex diff = c1.subtract(c2);
System.out.println("Difference between C1 and C2 is:"+diff.toString());
}
}
```
这段程序展示了如何利用之前编写的复数类执行一些基础的操作,比如相加与相减。
阅读全文
相关推荐
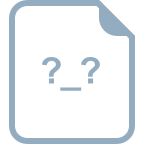
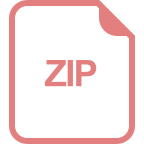
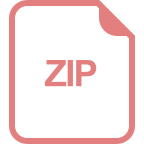
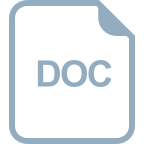
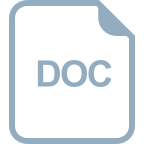
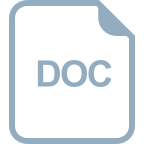
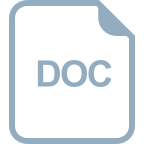
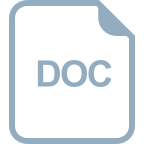
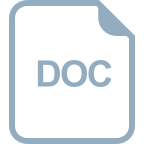
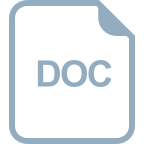
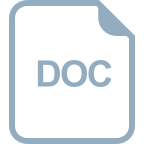
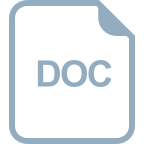
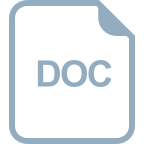
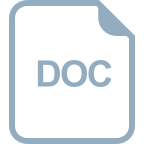
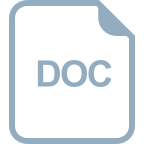
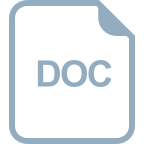
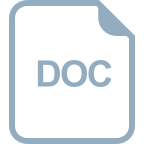
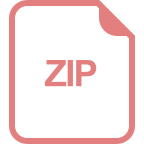
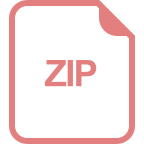