C语言 二维函数指针数组 详细示例
时间: 2024-12-11 08:06:20 浏览: 30
在C语言中,二维函数指针数组是一种高级特性,它允许我们存储一组指向函数的指针,每个函数接受不同的参数并返回不同类型的结果。这种结构通常用于回调函数、事件处理或其他需要动态调用函数的场景。
以下是一个简单的二维函数指针数组的示例:
```c
#include <stdio.h>
// 定义三个函数原型作为我们的函数指针类型
typedef int (*func1)(int);
typedef double (*func2)(double);
typedef char (*func3)(char);
// 函数指针数组
func1 funcArray1[2][2] = {
{addIntegers, multiplyIntegers},
{addDoubles, multiplyDoubles}
};
func2 funcArray2[2][2] = {
{addIntegersToDoubles, subtractIntegersFromDoubles},
{divideDoubles, multiplyDoubles}
};
func3 funcArray3[2][2] = {
{addChars, compareChars},
{concatenateChars, printChar}
};
// 定义实际函数
int addIntegers(int a, int b) {
return a + b;
}
double multiplyIntegers(int a, int b) {
return (double)a * b;
}
double addIntegersToDoubles(double a, int b) {
return a + (double)b;
}
//...其他函数...
// 使用函数指针数组
void processArray(func1 array[], int x, int y) {
printf("Result of %d + %d is: %d\n", x, y, array[x][y]());
}
void main() {
// 调用相应的函数指针
processArray(funcArray1, 0, 0); // 示例调用 addIntegers(1, 2)
processArray(funcArray2, 0, 1); // 示例调用 addIntegersToDoubles(1.5, 2)
// 其他维度和函数类型的调用类似
}
```
在这个例子中,`funcArray1` 包含两个元素,每个元素都是一个大小为 2 的一维数组,分别存储 `addIntegers` 和 `multiplyIntegers` 这两个整数相加减的函数指针。通过传入对应的行和列索引,我们可以动态地选择并调用函数。
阅读全文
相关推荐
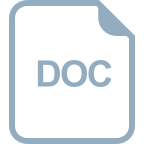
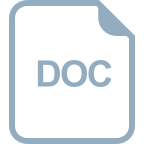
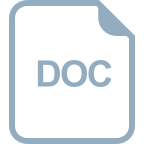

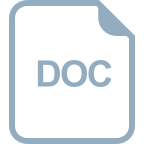
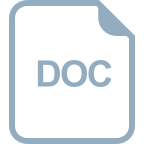
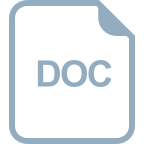
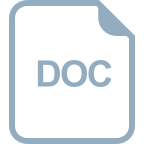
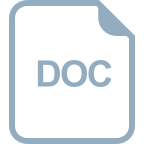




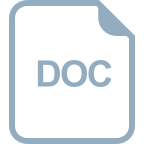
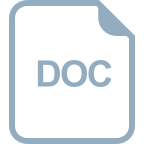
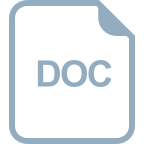
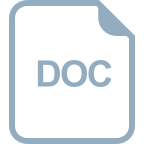
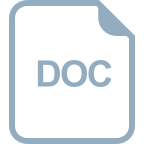
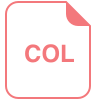