typescript输出语句
时间: 2024-04-22 08:19:23 浏览: 73
在TypeScript中,可以使用console.log()函数来输出语句。console.log()函数用于将消息打印到控制台。以下是一些示例:
1. 输出字符串:
console.log("Hello, TypeScript!");
2. 输出变量的值:
let num: number = 10;
console.log("The value of num is: " + num);
3. 输出表达式的结果:
let x: number = 5;
let y: number = 3;
console.log("The sum of x and y is: " + (x + y));
4. 输出对象:
let person = { name: "John", age: 30 };
console.log(person);
相关问题
typescript的switch语句怎么用
TypeScript的switch语句与JavaScript的用法相同,其语法如下所示:
```
switch(expression) {
case constant-expression:
statement(s);
break;
case constant-expression:
statement(s);
break;
// 可以有任意数量的 case 语句
default:
statement(s);
}
```
其中,expression是要进行比较的表达式,constant-expression是要比较的常量表达式,statement(s)是要执行的语句块。在TypeScript中,switch语句的expression可以是任何类型,而不仅仅是整型或枚举类型。
下面是一个TypeScript的switch语句的例子:
```typescript
let num: number = 2;
switch(num) {
case 1:
console.log("num is 1");
break;
case 2:
console.log("num is 2");
break;
default:
console.log("num is not 1 or 2");
}
```
在这个例子中,我们定义了一个变量num,并将其赋值为2。然后,我们使用switch语句来比较num的值。由于num的值为2,因此第二个case语句将被执行,输出"num is 2"。
用自己原创的语句详细说一下基于SpringBoot,TypeScript,Vue3健身房管理系统的输入输出格式设计,要求必须写出具体哪些内容输入,哪些输出
基于SpringBoot, TypeScript, Vue3的健身房管理系统的输入输出格式设计如下:
输入格式设计:
1. 用户登录输入格式
请求方式:POST
请求URL:/api/user/login
请求参数:
| 参数名 | 类型 | 是否必选 | 说明 |
| -------- | ------ | -------- | -------- |
| username | string | 是 | 用户名 |
| password | string | 是 | 用户密码 |
2. 用户注册输入格式
请求方式:POST
请求URL:/api/user/register
请求参数:
| 参数名 | 类型 | 是否必选 | 说明 |
| -------- | ------ | -------- | -------- |
| username | string | 是 | 用户名 |
| password | string | 是 | 用户密码 |
| phone | string | 是 | 手机号码 |
| email | string | 是 | 邮箱地址 |
3. 添加健身房设备输入格式
请求方式:POST
请求URL:/api/gym/equipment/add
请求参数:
| 参数名 | 类型 | 是否必选 | 说明 |
| -------------- | ------- | -------- | ---------------- |
| name | string | 是 | 设备名称 |
| type | string | 是 | 设备类型 |
| price | number | 是 | 设备价格 |
| inventory | number | 是 | 设备库存 |
| purchase_date | string | 是 | 设备购买日期 |
| manufacturer | string | 是 | 设备生产厂家 |
| instruction | string | 是 | 设备使用说明书 |
| maintenance | string | 是 | 设备维护保养说明 |
| warranty | string | 是 | 设备保修说明 |
| picture_url | string | 是 | 设备图片URL |
| supplier_phone | string | 是 | 设备供应商电话 |
4. 添加健身房会员输入格式
请求方式:POST
请求URL:/api/gym/member/add
请求参数:
| 参数名 | 类型 | 是否必选 | 说明 |
| ------------ | ------ | -------- | ------------ |
| name | string | 是 | 会员姓名 |
| age | number | 是 | 会员年龄 |
| sex | string | 是 | 会员性别 |
| phone | string | 是 | 会员电话 |
| email | string | 是 | 会员邮箱 |
| registerDate | string | 是 | 会员注册日期 |
| remarks | string | 是 | 备注信息 |
5. 添加健身房教练输入格式
请求方式:POST
请求URL:/api/gym/coach/add
请求参数:
| 参数名 | 类型 | 是否必选 | 说明 |
| ------------ | ------ | -------- | ------------ |
| name | string | 是 | 教练姓名 |
| age | number | 是 | 教练年龄 |
| sex | string | 是 | 教练性别 |
| phone | string | 是 | 教练电话 |
| email | string | 是 | 教练邮箱 |
| hireDate | string | 是 | 教练入职日期 |
| salary | number | 是 | 教练薪资 |
| introduction | string | 是 | 教练简介 |
输出格式设计:
1. 用户登录成功输出格式
返回数据:
| 参数名 | 类型 | 说明 |
| --------- | ------ | -------- |
| token | string | 用户令牌 |
| username | string | 用户名 |
| avatarUrl | string | 用户头像 |
2. 用户注册成功输出格式
返回数据:
| 参数名 | 类型 | 说明 |
| -------- | ------ | -------- |
| username | string | 用户名 |
| phone | string | 手机号码 |
| email | string | 邮箱地址 |
3. 添加健身房设备成功输出格式
返回数据:
| 参数名 | 类型 | 说明 |
| ------ | ------ | -------- |
| name | string | 设备名称 |
| type | string | 设备类型 |
| price | number | 设备价格 |
4. 添加健身房会员成功输出格式
返回数据:
| 参数名 | 类型 | 说明 |
| ------ | ------ | -------- |
| name | string | 会员姓名 |
| age | number | 会员年龄 |
5. 添加健身房教练成功输出格式
返回数据:
| 参数名 | 类型 | 说明 |
| ------ | ------ | -------- |
| name | string | 教练姓名 |
| age | number | 教练年龄 |
| salary | number | 教练薪资 |
阅读全文
相关推荐
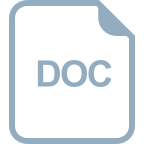
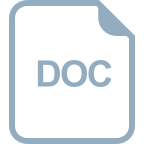
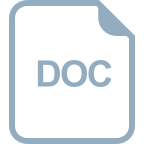
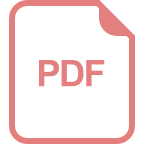
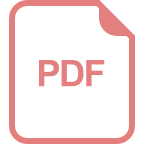
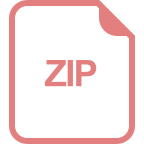
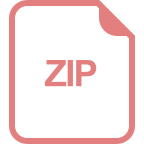
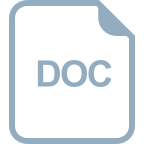
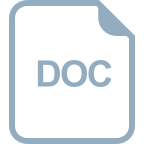
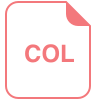
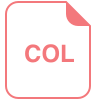
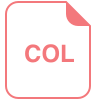
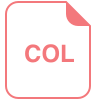



