python自动下载网页附件中的word和excel文件
时间: 2024-09-26 08:07:10 浏览: 6
在Python中,你可以使用一些库来自动化下载网页附件中的Word和Excel文件,如`requests`, `BeautifulSoup`用于抓取网页内容,以及`pandas`和`openpyxl`处理Excel文件,`python-docx`处理Word文档。以下是简单的步骤:
1. **安装必要的库**:
- 安装`requests`: `pip install requests`
- 安装`beautifulsoup4`: `pip install beautifulsoup4`
- 对于处理Excel和Word文档,还需要安装`pandas`, `openpyxl`, 和 `python-docx`:
```
pip install pandas openpyxl python-docx
```
2. **编写脚本**:
```python
import os
import requests
from bs4 import BeautifulSoup
import pandas as pd
from docx import Document
def download_files(url):
# 使用requests获取网页内容
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 找到所有需要下载的链接(这取决于HTML结构)
file_links = soup.select('.file-link') # 例如,假设目标文件在一个class为"file-link"的元素下
for link in file_links:
filename = link.get('href').split('/')[-1] # 获取文件名
extension = filename.split('.')[-1] # 获取文件扩展名
if extension in ['doc', 'docx'] or extension == 'xlsx': # 检查是否是Word或Excel
# 如果是Word文档
if extension in ['doc', 'docx']:
r = requests.get(link['href'])
with open(filename, 'wb') as f:
f.write(r.content)
# 如果是Excel文件
elif extension == 'xlsx':
r = requests.get(link['href'])
data = pd.read_excel(io=r.content) # 先读取内容再保存
df_path = os.path.join(os.getcwd(), filename)
data.to_excel(df_path, index=False)
url_to_download = "http://example.com" # 替换为实际网页URL
download_files(url_to_download)
```
3. **注意**:
- 你需要根据实际网页的HTML结构来修改`file_links`的选择器。
- 文件路径可以按照需要自定义,这里使用了当前工作目录。
相关推荐
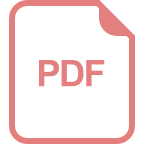
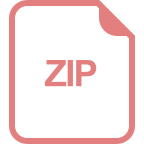
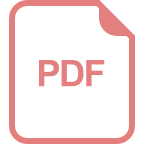
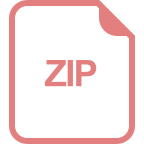
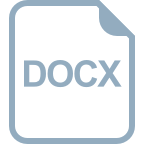
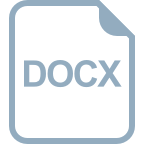
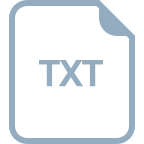
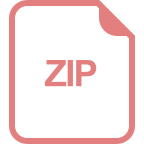
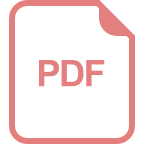
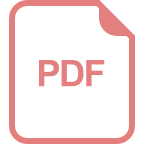
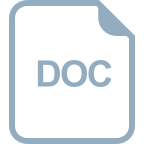
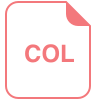





