python if else continue
时间: 2024-01-10 20:20:45 浏览: 226
在Python中,if-else语句用于根据条件执行不同的代码块。如果条件为True,则执行if代码块;如果条件为False,则执行else代码块。continue语句用于跳过当前循环中的剩余代码,并继续下一次循环。
以下是一个示例,演示了if-else和continue的使用:
```python
name = input("Please input your name: ")
if name == "Johnson":
print("Hello my son.")
else:
print("Hello my friend.")
for i in range(1, 6):
if i == 3:
continue
print(i)
```
运行上述代码,根据输入的名字,程序将打印不同的问候语。如果输入的名字是"Johnson",则打印"Hello my son.",否则打印"Hello my friend."。接下来,使用for循环打印数字1到5,但是当数字等于3时,使用continue语句跳过该次循环。
相关问题
if continue else语句
if-continue-else语句是一种在编程中常用的条件控制语句,用于根据条件执行不同的代码块。它的基本语法如下:
```
if condition:
# 如果条件为真,则执行这里的代码
continue
else:
# 如果条件为假,则执行这里的代码
```
在这个语法中,`condition`是一个表达式,它的值可以是True或False。如果`condition`为True,那么if语句块中的代码会被执行,然后跳过后续的代码继续执行循环的下一次迭代。如果`condition`为False,那么else语句块中的代码会被执行。
`continue`关键字用于跳过当前循环迭代中剩余的代码,直接进入下一次迭代。它通常与循环语句(如for循环或while循环)一起使用。
下面是一个示例,演示了if-continue-else语句的用法:
```python
numbers = [1, 2, 3, 4, 5]
for num in numbers:
if num % 2 == 0:
continue
else:
print(num)
# 输出结果:
# 1
# 3
# 5
```
在上面的示例中,我们使用for循环遍历了一个列表`numbers`。对于列表中的每个元素,如果它是偶数,则使用`continue`跳过后续的代码,直接进入下一次循环迭代。如果它是奇数,则执行`else`语句块中的代码,打印出该奇数。
python if
In Python, `if` is a conditional statement that allows you to execute a block of code only if a certain condition is met. Here's the basic syntax of an `if` statement:
```python
if condition:
# code to execute if condition is True
```
The `condition` can be any expression that evaluates to a Boolean value (`True` or `False`). If the `condition` is `True`, the code block indented under the `if` statement will be executed. If the `condition` is `False`, the code block will be skipped and execution will continue with the next statement after the `if` block.
You can also use an `else` statement to specify a block of code to execute if the `condition` is `False`:
```python
if condition:
# code to execute if condition is True
else:
# code to execute if condition is False
```
Finally, you can use an `elif` statement (short for "else if") to specify additional conditions to check:
```python
if condition1:
# code to execute if condition1 is True
elif condition2:
# code to execute if condition2 is True and condition1 is False
else:
# code to execute if both condition1 and condition2 are False
```
Here's an example to help illustrate how `if` statements work:
```python
x = 5
if x > 0:
print("x is positive")
else:
print("x is non-positive")
```
In this example, the `condition` is `x > 0`. Since `x` is equal to `5`, which is greater than `0`, the code block under the `if` statement will be executed, and the output will be:
```
x is positive
```
I hope this helps! Let me know if you have any other questions.
阅读全文
相关推荐
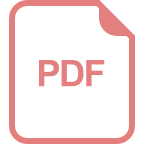

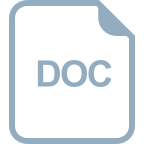
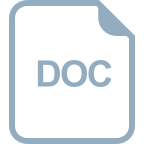
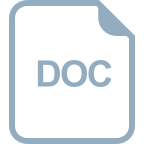
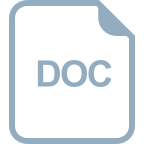
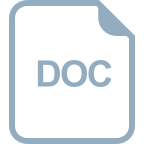
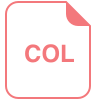






