用c++求整数数组中相差最小的两个元素(称为最接近数)的差值。 输入格式: 首先输入数组的长度n,2<=n<=10000,然后输入n个整数作为数组元素。 输出格式: 输出数组中相差最小的两个元素的差的绝对值。
时间: 2024-10-23 19:11:08 浏览: 26
在 C++ 中,可以使用排序算法(如快速排序、归并排序等)先对整数数组进行排序,然后再找到相邻的两个元素,它们之间的差即是最小的差值。以下是简单的步骤:
1. 输入数组长度 n 和数组元素。
2. 使用 `sort` 函数将数组按升序排列。
3. 初始化两个变量,分别存储第一个元素(最小的元素)和第二小的元素(最小的差),初始值设为数组的第一个元素和第二个元素。
4. 遍历已排序的数组,如果当前元素比第二小的元素还要小,更新第二小的元素;遍历完成后,两者的差值即为所求。
5. 输出结果:两元素之差的绝对值。
以下是一个简化的示例代码片段:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
int findClosestDifference(const std::vector<int>& arr) {
int n = arr.size();
if (n < 2) {
return -1; // 如果数组只有一个元素,无法找到差值
}
std::vector<int> sortedArr(arr);
std::sort(sortedArr.begin(), sortedArr.end());
int minDiff = abs(sortedArr[1] - sortedArr[0]);
for (int i = 2; i < n; i++) {
int diff = abs(sortedArr[i] - sortedArr[i - 1]);
if (diff < minDiff) {
minDiff = diff;
}
}
return minDiff;
}
int main() {
int n;
std::cout << "Enter the length of the array: ";
std::cin >> n;
std::vector<int> arr(n);
std::cout << "Enter array elements: ";
for (int& num : arr) {
std::cin >> num;
}
int result = findClosestDifference(arr);
std::cout << "The difference between the closest numbers is: " << result << std::endl;
return 0;
}
```
阅读全文
相关推荐
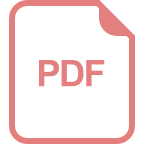
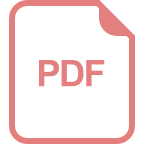
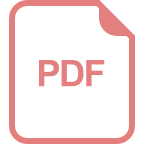


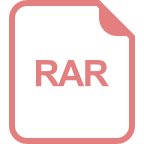
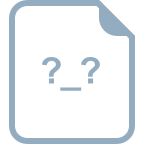
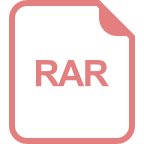
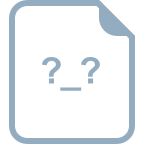
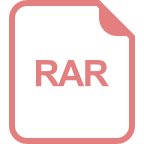
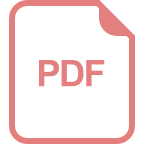
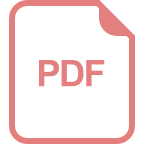
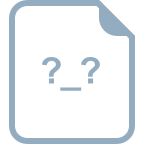
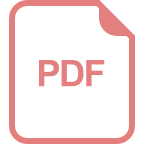
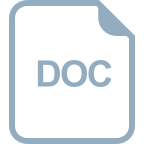
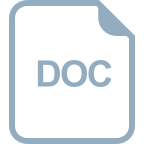