按照2000.11.1 12:00~18:00这种格式输入并存储多个时间数据
时间: 2024-10-01 15:09:18 浏览: 53
在C++中,为了按照"2000.11.1 12:00~18:00"这样的格式输入并存储多个时间数据,我们可以使用`std::chrono`库结合`std::string`来处理。首先,我们需要定义一个自定义的时间区间类,包含开始时间和结束时间。下面是一个简单的示例:
```cpp
#include <iostream>
#include <vector>
#include <string>
#include <chrono>
#include <sstream>
class TimeInterval {
public:
using clock_type = std::chrono::system_clock;
std::chrono::time_point<clock_type> start;
std::chrono::time_point<clock_type> end;
TimeInterval(const std::string& str) {
parse(str);
}
private:
void parse(const std::string& input) {
auto parts = input.split(' ');
start = parseTime(parts[0]);
if (parts.size() > 1 && parts[1].find('~') != std::string::npos) {
auto split_range = parts[1].split('~');
end = parseTime(split_range[1]);
} else {
end = start + std::chrono::hours(6); // 假设如果没有特殊字符,默认持续6个小时
}
}
std::chrono::time_point<clock_type> parseTime(const std::string& time_str) {
struct tm parsed_time;
std::istringstream ss(time_str);
ss >> std::get_time(&parsed_time, "%Y.%m.%d %H:%M");
return clock_type::from_time_t(std::mktime(&parsed_time));
}
// 自定义的分割字符串功能,这里仅做演示,实际应用可能需要第三方库
std::vector<std::string> split(const std::string& s, char delimiter) {
std::vector<std::string> tokens;
size_t pos = 0;
std::string token;
while ((pos = s.find(delimiter)) != std::string::npos) {
token = s.substr(0, pos);
tokens.push_back(token);
s.erase(0, pos + 1);
}
if (!s.empty()) {
tokens.push_back(s);
}
return tokens;
}
};
int main() {
std::vector<TimeInterval> timeIntervals;
std::string input;
while (true) {
std::cout << "Enter a time interval in format 'YYYY.MM.DD HH:MM~HH:MM' (or 'q' to quit): ";
getline(std::cin, input);
if (input == "q") {
break;
}
timeIntervals.push_back(TimeInterval(input));
}
for (const auto& ti : timeIntervals) {
ti.print();
}
return 0;
}
// 自定义打印函数
void TimeInterval::print() const {
std::cout << "Start: " << start << ", End: " << end << std::endl;
}
阅读全文
相关推荐





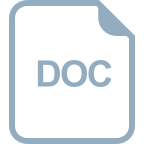
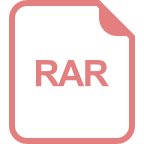











