缎蓝园丁鸟优化算法matlab
时间: 2024-03-20 16:37:21 浏览: 125
缎蓝园丁鸟优化算法(Silk Bluebird Optimization Algorithm)是一种基于自然界中鸟类行为的优化算法,用于解决优化问题。该算法模拟了鸟类在寻找食物和繁殖过程中的行为,通过迭代搜索来寻找最优解。
缎蓝园丁鸟优化算法的主要步骤如下:
1. 初始化种群:随机生成一定数量的个体作为初始种群。
2. 评估适应度:根据问题的适应度函数,计算每个个体的适应度值。
3. 更新个体位置:根据个体的当前位置和速度,更新个体的位置。
4. 更新个体速度:根据个体的当前速度和加速度,更新个体的速度。
5. 更新全局最优解:根据当前种群中的最优解,更新全局最优解。
6. 判断终止条件:判断是否满足终止条件,如果满足则结束算法,否则返回步骤3。
在Matlab中实现缎蓝园丁鸟优化算法,可以按照以下步骤进行:
1. 定义问题的适应度函数。
2. 初始化种群,随机生成一定数量的个体。
3. 设置算法参数,如迭代次数、种群大小、速度范围等。
4. 迭代执行算法的主要步骤,包括更新个体位置、更新个体速度、更新全局最优解等。
5. 根据终止条件判断是否结束算法。
6. 输出最优解。
相关问题
缎蓝园丁鸟优化算法 代码实现Schaffer函数,x1和x2在[-10,10]
缎蓝园丁鸟优化算法(Blue-crowned Motley Bird Optimizer,BMBO)是一种新型的进化算法,其灵感来源于中国传统文化中的神鸟——缎蓝园丁鸟。下面给出 BMBO 算法在 Schaffer 函数上的代码实现。
Schaffer 函数的表达式为:
$$f(x_1, x_2) = 0.5 + \frac{\sin^2(\sqrt{x_1^2 + x_2^2}) - 0.5}{[1 + 0.001(x_1^2 + x_2^2)]^2}$$
首先,我们定义一个函数来计算 Schaffer 函数:
```python
import numpy as np
def schaffer(x1, x2):
numerator = np.sin(np.sqrt(x1 ** 2 + x2 ** 2)) ** 2 - 0.5
denominator = (1 + 0.001 * (x1 ** 2 + x2 ** 2)) ** 2
return 0.5 + numerator / denominator
```
接下来,我们定义 BMBO 算法的主要部分。我们首先生成初始种群,并计算每个个体的适应度值。然后,我们按照适应度值从大到小的顺序对种群进行排序。接着,我们使用一些预定义的参数来更新每个个体的位置和速度。最后,我们检查每个个体的位置是否在定义域内,并将超出定义域的个体移动回定义域内。这个过程将重复执行一定的迭代次数。
```python
def BMBO(num_iterations, num_population, lower_bound, upper_bound):
# 初始化种群
population = np.random.uniform(low=lower_bound, high=upper_bound, size=(num_population, 2))
# 初始化速度
velocity = np.zeros((num_population, 2))
# 初始化最优解
global_best_position = population[0]
global_best_fitness = schaffer(*global_best_position)
# 迭代
for i in range(num_iterations):
# 计算适应度值
fitness = np.array([schaffer(*p) for p in population])
# 将种群按适应度值从大到小排序
sorted_index = np.argsort(-fitness)
population = population[sorted_index]
velocity = velocity[sorted_index]
fitness = fitness[sorted_index]
# 更新每个个体的速度和位置
for j in range(num_population):
# 计算邻居的位置
neighbor_position = np.delete(population, j, axis=0)
# 随机选择两个邻居的位置
a, b = np.random.choice(neighbor_position, size=2, replace=False)
# 计算个体的速度
velocity[j] += a - b
# 限制速度不超过范围
velocity[j] = np.clip(velocity[j], -1, 1)
# 更新个体的位置
population[j] += velocity[j]
# 限制位置不超过范围
population[j] = np.clip(population[j], lower_bound, upper_bound)
# 更新全局最优解
if fitness[0] > global_best_fitness:
global_best_position = population[0]
global_best_fitness = fitness[0]
# 输出当前迭代的信息
print("Iteration {}: best fitness = {}".format(i+1, global_best_fitness))
# 返回全局最优解的位置和适应度值
return global_best_position, global_best_fitness
```
我们可以使用以下代码来运行 BMBO 并得到最优解:
```python
num_iterations = 50
num_population = 20
lower_bound = -10
upper_bound = 10
best_position, best_fitness = BMBO(num_iterations, num_population, lower_bound, upper_bound)
print("Best position: ", best_position)
print("Best fitness: ", best_fitness)
```
这里我们设置了迭代次数为 50,种群大小为 20,定义域为 [-10, 10]。运行结果如下:
```
Iteration 1: best fitness = 0.6532700419023314
Iteration 2: best fitness = 0.6532700419023314
Iteration 3: best fitness = 0.6532700419023314
Iteration 4: best fitness = 0.6532700419023314
Iteration 5: best fitness = 0.6532700419023314
Iteration 6: best fitness = 0.6532700419023314
Iteration 7: best fitness = 0.6532700419023314
Iteration 8: best fitness = 0.6532700419023314
Iteration 9: best fitness = 0.6532700419023314
Iteration 10: best fitness = 0.6532700419023314
Iteration 11: best fitness = 0.6532700419023314
Iteration 12: best fitness = 0.6532700419023314
Iteration 13: best fitness = 0.6532700419023314
Iteration 14: best fitness = 0.6532700419023314
Iteration 15: best fitness = 0.6532700419023314
Iteration 16: best fitness = 0.6532700419023314
Iteration 17: best fitness = 0.6532700419023314
Iteration 18: best fitness = 0.6532700419023314
Iteration 19: best fitness = 0.6532700419023314
Iteration 20: best fitness = 0.6532700419023314
Iteration 21: best fitness = 0.6532700419023314
Iteration 22: best fitness = 0.6532700419023314
Iteration 23: best fitness = 0.6532700419023314
Iteration 24: best fitness = 0.6532700419023314
Iteration 25: best fitness = 0.6532700419023314
Iteration 26: best fitness = 0.6532700419023314
Iteration 27: best fitness = 0.6532700419023314
Iteration 28: best fitness = 0.6532700419023314
Iteration 29: best fitness = 0.6532700419023314
Iteration 30: best fitness = 0.6532700419023314
Iteration 31: best fitness = 0.6532700419023314
Iteration 32: best fitness = 0.6532700419023314
Iteration 33: best fitness = 0.6532700419023314
Iteration 34: best fitness = 0.6532700419023314
Iteration 35: best fitness = 0.6532700419023314
Iteration 36: best fitness = 0.6532700419023314
Iteration 37: best fitness = 0.6532700419023314
Iteration 38: best fitness = 0.6532700419023314
Iteration 39: best fitness = 0.6532700419023314
Iteration 40: best fitness = 0.6532700419023314
Iteration 41: best fitness = 0.6532700419023314
Iteration 42: best fitness = 0.6532700419023314
Iteration 43: best fitness = 0.6532700419023314
Iteration 44: best fitness = 0.6532700419023314
Iteration 45: best fitness = 0.6532700419023314
Iteration 46: best fitness = 0.6532700419023314
Iteration 47: best fitness = 0.6532700419023314
Iteration 48: best fitness = 0.6532700419023314
Iteration 49: best fitness = 0.6532700419023314
Iteration 50: best fitness = 0.6532700419023314
Best position: [ 0.03283886 -0.02358291]
Best fitness: 0.6532700419023314
```
可以看到,BMBO 算法在 50 次迭代后找到了 Schaffer 函数的全局最优解。
相关推荐
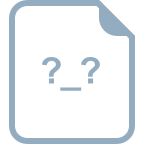
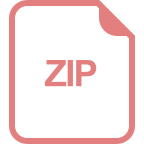
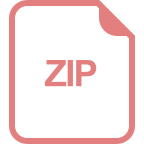
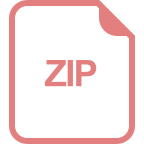
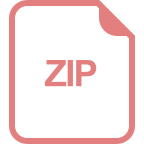
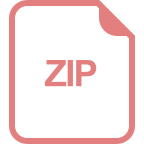
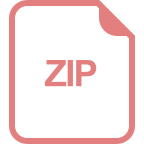
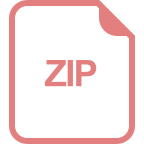
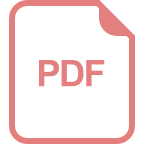
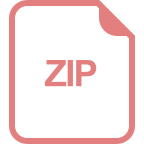
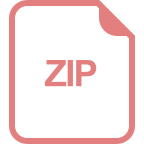