python BFS框架
时间: 2023-09-06 09:12:10 浏览: 52
一个常见的Python BFS(广度优先搜索)框架可以如下所示:
```python
from collections import deque
def bfs(graph, start):
visited = set() # 用于记录已访问过的节点
queue = deque([start]) # 使用双端队列作为搜索队列
while queue:
node = queue.popleft() # 从队列左侧取出一个节点
if node not in visited:
visited.add(node)
# 处理节点
print(node)
# 将该节点的未访问邻居加入队列
for neighbor in graph[node]:
if neighbor not in visited:
queue.append(neighbor)
```
在这个框架中,我们使用了一个`visited`集合来记录已访问过的节点,并使用双端队列`deque`作为搜索队列。我们从起始节点开始,不断从队列的左侧取出节点进行处理,并将其未访问过的邻居加入队列。这样可以保证节点按照广度优先的顺序进行访问。
你可以根据具体的问题,将上述框架进行适当修改和扩展。
相关问题
python BFS
BFS是广度优先搜索的缩写,是一种图的遍历算法,用于在图或树中按照层级进行搜索。BFS从给定的起始节点开始,逐层遍历节点,直到找到目标节点或遍历完整个图。BFS的原理是"逐层扩散",从起点出发,按层次从近到远,逐层先后搜索。在编码实现上,可以使用队列来实现BFS算法。BFS一般用于求解最短路径问题,因为BFS的特点是逐层搜索,先搜到的层离起点更近。在Python中,可以使用队列数据结构和循环来实现BFS算法。
#### 引用[.reference_title]
- *1* [python广度优先搜索(BFS)从入门到精通](https://blog.csdn.net/meridian002/article/details/131333459)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [Python算法:BFS](https://blog.csdn.net/qq_65144447/article/details/129048917)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
python bfs
BFS(广度优先搜索)是一种图遍历算法,用于解决图上的问题。在Python中,可以使用队列来实现BFS。引用和引用中的代码示例展示了如何使用BFS遍历树节点的层级。
在代码示例中,首先需要根据给定的树结构创建一个队列,并将根节点入队。然后,使用一个循环来迭代处理队列中的节点。在每次循环中,先获取当前队列的长度n,然后创建一个临时列表temp用于存储当前层级的节点值。
接下来,使用一个for循环遍历当前层级的节点数n,依次从队列中弹出节点a,并将其值a.val添加到临时列表temp中。然后,将该节点的左右子节点(如果存在)加入队列中,以便在下一次迭代中处理。
最后,在每一次循环结束后,将临时列表temp添加到结果列表res中,表示当前层级的节点值已经遍历完毕。当队列为空时,表示已经遍历完整个树,可以返回结果列表res作为最终结果。
需要注意的是,由于队列是先进先出的特性,所以在遍历每一层节点时,可以根据需要选择是否需要翻转临时列表temp来保证节点值的顺序。引用中的示例代码展示了如何使用BFS遍历图。
总结起来,使用BFS可以实现对树节点的层级遍历,通过队列来辅助实现节点的入队和出队操作。每一次从队列中取出节点时,将其子节点加入队列以便后续遍历。这样就可以按照层级顺序遍历树的节点,并将节点值保存到结果列表中返回。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [广度优先算法(BFS)-python](https://blog.csdn.net/weixin_44571635/article/details/123891023)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 50%"]
- *2* [基于python模拟bfs和dfs代码实例](https://download.csdn.net/download/weixin_38549721/14839350)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
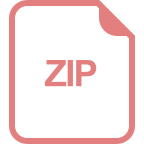
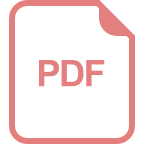












