1、完成本PPT中的第一个Servlet示例的代码编写,运行和调试。 在Servlet中覆盖init()方法,service()方法,destroy()方法;运行并实验这三个方法的调用时间点和调用次数。
时间: 2024-10-25 19:08:42 浏览: 33
首先,你需要了解在Servlet开发中,`javax.servlet.http.HttpServlet` 是最基础的Servlet类,它并没有提供自定义的`init()`, `service()` 和 `destroy()` 方法。但在实际应用中,我们可以创建一个继承自`HttpServlet` 的自定义Servlet,并覆盖这些方法:
```java
import javax.servlet.*;
import java.io.*;
public class CustomServlet extends HttpServlet {
private int initCount = 0;
private int serviceCount = 0;
private boolean destroyed = false;
@Override
public void init(ServletConfig config) throws ServletException {
System.out.println("CustomServlet.init() is called.");
initCount++;
super.init(config);
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("CustomServlet.service() is called (doGet).");
serviceCount++;
// Your logic here
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("CustomServlet.service() is called (doPost).");
serviceCount++;
// Your logic here
}
@Override
public void destroy() {
System.out.println("CustomServlet.destroy() is called.");
destroyed = true;
initCount = 0; // Reset counts after destruction
super.destroy();
}
public static void main(String[] args) {
try {
ServletContext context = new MockServletContext(); // Use a mock context for testing
CustomServlet servlet = new CustomServlet();
context.addServlet("customServlet", servlet);
context.getRequestDispatcher("/your-path").forward(request, response); // Simulate a request to trigger the methods
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在这个例子中,`init()`会在服务器启动时调用一次,`service()`会在处理每个HTTP请求时分别调用`doGet()` 或 `doPost()`,而`destroy()`会在Web应用卸载时调用。
你可以通过`main`方法测试这个Servlet,发送一个GET或POST请求到指定路径(例如`/your-path`),观察控制台的日志输出,以确认方法的调用情况。
阅读全文
相关推荐
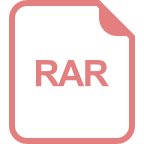
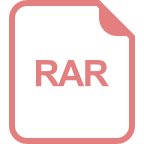
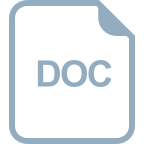
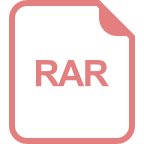
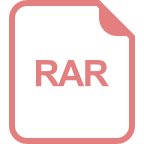
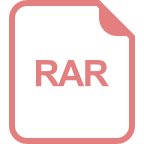
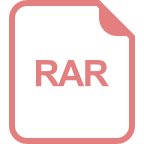
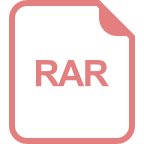
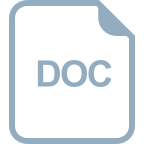
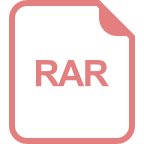
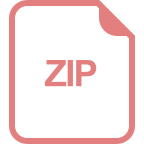
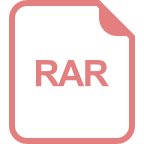
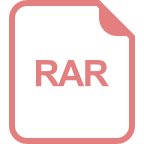
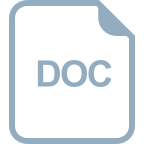
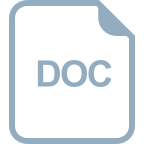
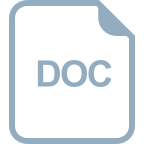
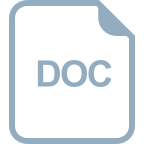
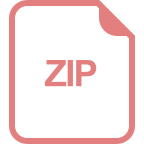