winform 消息队列
时间: 2023-12-05 20:05:14 浏览: 261
WinForm 消息队列是一个用于存储和处理 Windows 消息的队列。在 WinForm 应用程序中,所有的用户交互事件(如鼠标点击、键盘输入等)都会被转换成 Windows 消息,并被发送到消息队列中。消息队列会按照先进先出的顺序处理这些消息,并将它们分发给相应的窗口或控件进行处理。
WinForm 消息队列的主要作用是协调和管理应用程序中的各种事件和操作,确保它们能够按照正确的顺序被处理。例如,当用户点击一个按钮时,按钮控件会将一个 WM_CLICK 消息发送到消息队列中,然后消息队列会将这个消息分发给相应的窗口或控件进行处理。
WinForm 消息队列还可以用于实现异步操作和线程间通信。通过将自定义消息发送到消息队列中,不同的线程可以进行通信和协作,从而实现复杂的应用程序逻辑。
相关问题
c# winform IBM mq消息队列监听代码
以下是一个简单的 C# WinForm IBM MQ 消息队列监听代码示例:
```csharp
using IBM.WMQ;
using System;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace IBM_MQ_Listener
{
public partial class Form1 : Form
{
private MQQueueManager queueManager;
private MQQueue queue;
private MQMessage message;
private MQGetMessageOptions options;
public Form1()
{
InitializeComponent();
}
private async void btnStart_Click(object sender, EventArgs e)
{
try
{
// 设置 IBM MQ 连接信息
var properties = new Hashtable();
properties.Add(MQC.TRANSPORT_PROPERTY, MQC.TRANSPORT_MQSERIES_MANAGED);
properties.Add(MQC.HOST_NAME_PROPERTY, "your.mq.server.ip");
properties.Add(MQC.CHANNEL_PROPERTY, "your.mq.channel");
properties.Add(MQC.PORT_PROPERTY, your.mq.server.port);
properties.Add(MQC.USER_ID_PROPERTY, "your.mq.user");
properties.Add(MQC.PASSWORD_PROPERTY, "your.mq.password");
// 连接 IBM MQ 队列管理器
queueManager = new MQQueueManager("your.mq.queue.manager.name", properties);
// 打开监听的队列
queue = queueManager.AccessQueue("your.mq.queue.name", MQC.MQOO_INPUT_AS_Q_DEF + MQC.MQOO_FAIL_IF_QUIESCING);
// 初始化消息和获取选项
message = new MQMessage();
options = new MQGetMessageOptions();
// 启动监听循环
await Task.Run(() =>
{
while (true)
{
try
{
queue.Get(message, options);
// 处理接收到的消息
string msgText = message.ReadString(message.MessageLength);
Invoke(new Action(() => {
// 在 UI 线程中更新 UI
txtReceivedMsg.AppendText(msgText + Environment.NewLine);
}));
}
catch (MQException ex)
{
if (ex.ReasonCode == MQC.MQRC_NO_MSG_AVAILABLE)
{
// 没有消息可用,继续循环
continue;
}
else
{
// 其他错误,退出循环
throw ex;
}
}
}
});
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
private void btnStop_Click(object sender, EventArgs e)
{
try
{
// 关闭队列
if (queue != null)
{
queue.Close();
}
// 断开队列管理器连接
if (queueManager != null)
{
queueManager.Disconnect();
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
}
}
```
在这个示例中,我们使用 IBM.WMQ 库来连接 IBM MQ 队列管理器,打开监听的队列,接收并处理消息。需要注意的是,IBM.WMQ 库需要安装 IBM MQ 客户端才能正常使用。
写一段c# winform程序 IBM mq消息队列监听消息的代码
好的,以下是一段 C# WinForm 程序监听 IBM MQ 消息队列的代码:
```csharp
using IBM.WMQ;
using System;
using System.Windows.Forms;
namespace MQListener
{
public partial class Form1 : Form
{
MQQueueManager queueManager = null;
MQQueue queue = null;
MQMessage message = null;
MQGetMessageOptions options = null;
bool isListening = false;
public Form1()
{
InitializeComponent();
}
private void btnConnect_Click(object sender, EventArgs e)
{
try
{
// 设置连接参数
MQEnvironment.Hostname = txtHostname.Text.Trim();
MQEnvironment.Channel = txtChannel.Text.Trim();
MQEnvironment.Port = int.Parse(txtPort.Text.Trim());
MQEnvironment.UserId = txtUsername.Text.Trim();
MQEnvironment.Password = txtPassword.Text.Trim();
// 连接队列管理器
queueManager = new MQQueueManager(txtQueueManager.Text.Trim());
// 打开队列
queue = queueManager.AccessQueue(txtQueue.Text.Trim(), MQC.MQOO_INPUT_AS_Q_DEF + MQC.MQOO_FAIL_IF_QUIESCING);
// 初始化消息对象和获取消息的选项
message = new MQMessage();
options = new MQGetMessageOptions();
options.Options |= MQC.MQGMO_NO_SYNCPOINT;
options.Options |= MQC.MQGMO_WAIT;
options.WaitInterval = int.Parse(txtTimeout.Text.Trim()) * 1000;
// 设置监听标志
isListening = true;
// 启动监听线程
bgwListener.RunWorkerAsync();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
private void btnDisconnect_Click(object sender, EventArgs e)
{
try
{
// 停止监听
isListening = false;
// 关闭队列
if (queue != null)
{
queue.Close();
queue = null;
}
// 断开队列管理器
if (queueManager != null)
{
queueManager.Disconnect();
queueManager = null;
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
private void bgwListener_DoWork(object sender, System.ComponentModel.DoWorkEventArgs e)
{
while (isListening)
{
try
{
// 获取消息
if (queue != null)
{
queue.Get(message, options);
// 处理消息
string body = message.ReadString(message.MessageLength);
// TODO: 处理消息的逻辑
// 清空消息体
message.ClearMessage();
}
}
catch (Exception ex)
{
// 如果队列管理器已经断开,则停止监听
if (ex.Message.Contains("MQRC_Q_MGR_NOT_AVAILABLE") || ex.Message.Contains("MQRC_CONNECTION_BROKEN"))
{
isListening = false;
}
}
}
}
}
}
```
以上代码中,`btnConnect_Click` 方法用于连接 IBM MQ 队列管理器,并启动监听线程;`btnDisconnect_Click` 方法用于停止监听并断开连接;`bgwListener_DoWork` 方法是监听线程的主体,不断从队列中获取消息,然后处理消息。
请注意,以上代码仅供参考,并未进行完整测试,具体实现方式可能需要根据实际情况进行调整。
阅读全文
相关推荐



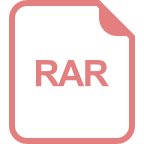








