vue3实现音频组件
时间: 2023-11-17 20:02:36 浏览: 100
Vue3可以通过使用`<audio>`标签来实现音频组件。在Vue3中,可以使用`ref`来获取`<audio>`标签的DOM元素,然后通过JavaScript来控制音频的播放、暂停、进度条等功能。
以下是一个简单的Vue3音频组件的示例代码:
```html
<template>
<div>
<audio ref="audio" :src="audioSrc"></audio>
<button @click="play">播放</button>
<button @click="pause">暂停</button>
<div>
<span>{{ currentTime }}</span>
<input type="range" :value="currentTime" :max="duration" @input="seek">
<span>{{ duration }}</span>
</div>
</div>
</template>
<script>
import { ref, computed, onMounted } from 'vue';
export default {
setup() {
const audioRef = ref(null);
const audioSrc = 'audio.mp3';
const currentTime = ref(0);
const duration = computed(() => {
return audioRef.value ? audioRef.value.duration : 0;
});
const play = () => {
audioRef.value.play();
};
const pause = () => {
audioRef.value.pause();
};
const seek = (event) => {
audioRef.value.currentTime = event.target.value;
};
const updateTime = () => {
currentTime.value = audioRef.value.currentTime;
};
onMounted(() => {
audioRef.value.addEventListener('timeupdate', updateTime);
});
return {
audioRef,
audioSrc,
currentTime,
duration,
play,
pause,
seek,
};
},
};
</script>
```
在上面的代码中,我们使用了Vue3的`ref`、`computed`和`onMounted`等API来实现音频组件。其中,`audioRef`是通过`ref`获取`<audio>`标签的DOM元素,`audioSrc`是音频文件的路径,`currentTime`是当前播放时间,`duration`是音频总时长。`play`、`pause`和`seek`分别是播放、暂停和拖动进度条的方法。`updateTime`是用来更新当前播放时间的方法,它会在音频播放时自动调用。
阅读全文
相关推荐
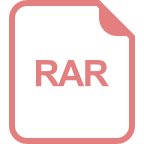
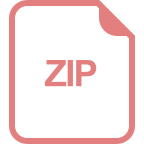
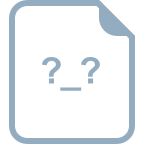
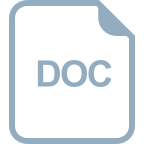
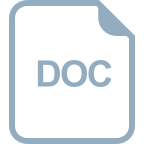



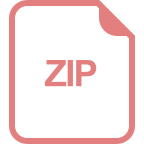
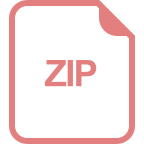
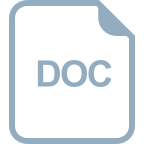




