function timeOut(){ return new Promise((resolve)=>{ setTimeout(()=>{ console.log("222") resolve() },1000) }) } async function test(){ console.log("111") await timeOut() console.log("333") } function test2(){ console.log("0000") test() console.log("4444") } test2()
时间: 2024-04-15 19:27:11 浏览: 127
根据给定的代码,我们可以分析出以下的输出结果:
1. 首先,调用 `test2()` 函数。
2. 输出结果为 `0000`,表示第一个 `console.log` 语句打印的内容。
3. 接着,调用 `test()` 函数。
4. 输出结果为 `111`,表示第二个 `console.log` 语句打印的内容。
5. 在 `test()` 函数中使用 `await` 等待 `timeOut()` 函数的执行结果。
6. `timeOut()` 函数返回一个 Promise 对象,在 1 秒后通过 `resolve()` 方法将 Promise 状态设置为已完成。
7. 在 1 秒后,`setTimeout` 回调函数中的 `console.log` 语句会打印出 `222`。
8. `timeOut()` 函数中的 Promise 对象状态变为已完成,继续执行下一行代码。
9. 输出结果为 `333`,表示第三个 `console.log` 语句打印的内容。
10. 回到 `test2()` 函数,继续执行下一行代码。
11. 输出结果为 `4444`,表示第四个 `console.log` 语句打印的内容。
因此,整个代码的输出结果是:
```
0000
111
222
333
4444
```
相关问题
function timeOut(){ return new Promise((resolve)=>{ setTimeout(()=>{ console.log("222") resolve() },1000) }) } async function test(){ console.log("111") await timeOut() console.log("333") } test()输出结果
根据给定的代码,`test()` 函数是一个异步函数,它使用了 `await` 关键字来等待 `timeOut()` 函数的执行结果。`timeOut()` 函数返回一个 Promise 对象,在 1 秒后通过 `resolve()` 方法将 Promise 状态设置为已完成。
因此,代码的输出结果如下:
1. 首先,调用 `test()` 函数。
2. 输出结果为 `111`,表示第一个 `console.log` 语句打印的内容。
3. 然后,`await` 关键字等待 `timeOut()` 函数的执行结果。
4. 在 1 秒后,`setTimeout` 回调函数中的 `console.log` 语句会打印出 `222`。
5. `timeOut()` 函数中的 Promise 对象状态变为已完成,继续执行下一行代码。
6. 最后,输出结果为 `333`,表示第二个 `console.log` 语句打印的内容。
因此,整个代码的输出结果是:
```
111
222
333
```
Given a function fn, an array or arguments args, and a timeout t in milliseconds, return a cancel function cancelFn. After a delay of t, fn should be called with args passed as parameters unless cancelFn was called first. In that case, fn should never be called. Example 1: Input: fn = (x) => x * 5, args = [2], t = 20, cancelTime = 50 Output: [{"time": 20, "returned": 10}] Explanation: const cancel = cancellable(fn, [2], 20); // fn(2) called at t=20ms setTimeout(cancel, 50); the cancelTime (50ms) is after the delay time (20ms), so fn(2) should be called at t=20ms. The value returned from fn is 10.
Here's one possible implementation of the `cancellable` function in JavaScript:
```
function cancellable(fn, args, t) {
let timerId;
const promise = new Promise((resolve, reject) => {
timerId = setTimeout(() => {
resolve(fn(...args));
}, t);
});
const cancelFn = () => {
clearTimeout(timerId);
promise.then(() => {
console.log("Function call cancelled");
});
};
return cancelFn;
}
```
The `cancellable` function takes three arguments: `fn`, the function to be called after a delay; `args`, an array of arguments to pass to `fn`; and `t`, the delay time in milliseconds.
The function returns a cancel function `cancelFn`, which can be called to cancel the delayed function call. If `cancelFn` is called before the delay time is up, `fn` will never be called and the promise returned from `cancellable` will be rejected.
In the example given, you would call `cancellable` like this:
```
const cancel = cancellable((x) => x * 5, [2], 20);
```
This sets up a delayed call to `fn`, which multiplies its argument by 5. The argument is 2, so the expected result is 10. The delay time is 20ms, and the cancel time is 50ms.
To cancel the delayed call, you would call `cancel()`:
```
setTimeout(cancel, 50);
```
This cancels the delayed call at the specified cancel time. Since the cancel time is after the delay time, `fn` will be called at t=20ms and will return the value 10.
阅读全文
相关推荐
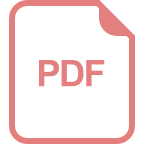
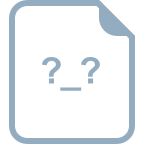
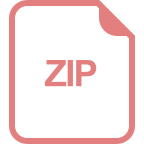
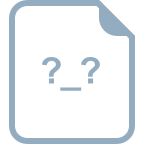
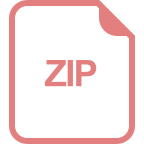
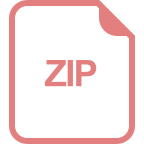
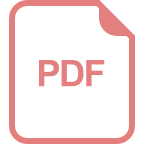
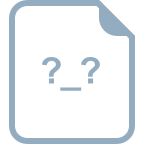
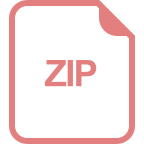
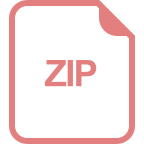
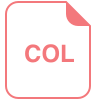





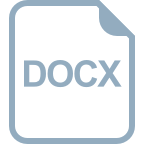