c语言正则表达式(1) 编写识别函数,该函数识别如下规则的字符串 规则: 1字符串长度在1到7之间; 2必须是英文和数字组成,英文大小写均可; 除编写识别函数外,需要提供对函数验证的主控代码
时间: 2024-10-24 10:04:16 浏览: 15
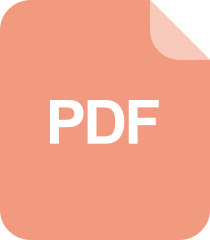
C语言中的正则表达式使用示例详解
在C语言中,虽然原生标准库并不直接支持正则表达式,但我们通常会借助一些外部库如PCRE (Perl Compatible Regular Expressions) 或者更现代的libregex来实现这个功能。这里我会给出一个简单的示例,首先你需要安装一个C正则表达式库,然后使用它来创建匹配规则。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "pcre.h" // 假设已经包含了pcre库
// 正则表达式模式
const char* regex_pattern = "^(?=.*[a-zA-Z])(?=.*\\d)[a-zA-Z0-9]{1,7}$";
// 函数原型,返回值类型为int,表示匹配结果
int is_valid_string(const char* input);
// 使用pcre库的匹配函数
int match_with_pcre(const char* pattern, const char* input) {
int rc;
PCRE* re = pcre_compile(pattern, 0, NULL, &rc, NULL);
if (rc != 0) {
fprintf(stderr, "Error compiling regex: %s\n", pcre_error_message(rc));
return -1;
}
int ovector[3]; // 匹配结果数组
rc = pcre_exec(re, NULL, input, strlen(input), 0, 0, ovector, 3);
pcre_free(re);
if (rc == 0 && ovector[1] == strlen(input)) {
// 匹配成功并且输入恰好被整个匹配
return 1;
} else {
return 0;
}
}
// 主函数
int main() {
const char* test_cases[] = { "abc123", "ab1cd", "abc1234567", "123", "aBcD_123" };
const size_t test_count = sizeof(test_cases) / sizeof(*test_cases);
for (size_t i = 0; i < test_count; i++) {
int isValid = is_valid_string(test_cases[i]);
printf("%s: %d\n", test_cases[i], isValid);
if (isValid)
printf("Regex matched: '%s'\n", test_cases[i]);
else
printf("Regex didn't match: '%s'\n", test_cases[i]);
}
return 0;
}
// 实现is_valid_string函数,调用match_with_pcre
int is_valid_string(const char* input) {
return match_with_pcre(regex_pattern, input);
}
阅读全文
相关推荐
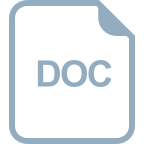
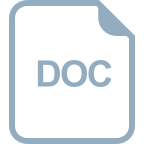
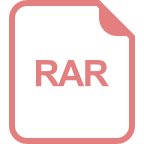
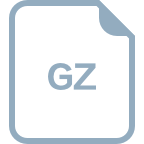
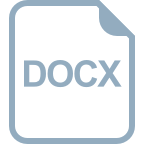
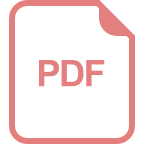
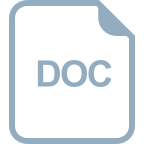
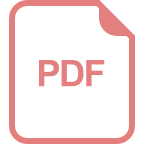
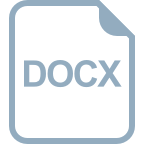
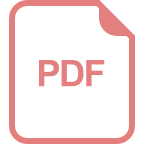
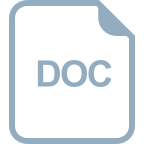
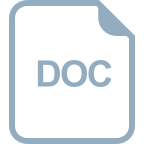
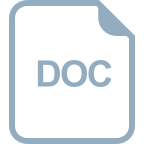




