c++ 用蛮力法计算n个三维坐标点的最近对坐标
时间: 2024-11-05 10:29:57 浏览: 31
在C++中,使用蛮力法(Brute Force)计算n个三维坐标点的最近对坐标意味着你需要遍历所有可能的配对,并找出两个点之间距离最小的一对。这是一个线性时间复杂度O(n^2)的算法,因为对于n个点,每个点都需要与其他n-1个点比较。
以下是一个简单的步骤说明:
1. 定义一个结构体或类`Point3D`来表示三维坐标,包含x、y、z三个属性。
```cpp
struct Point3D {
double x;
double y;
double z;
};
```
2. 创建一个函数来计算两点之间的欧氏距离(Euclidean distance):
```cpp
double distance(const Point3D& p1, const Point3D& p2) {
return sqrt(pow(p1.x - p2.x, 2) + pow(p1.y - p2.y, 2) + pow(p1.z - p2.z, 2));
}
```
3. 主函数中,初始化一个向量`points`存储所有的三维点,然后用嵌套循环找到最近的对:
```cpp
std::vector<Point3D> points; // 假设已填充好n个点
if (points.size() > 1) {
double minDistance = INFINITY;
std::pair<Point3D, Point3D> closestPair;
for (size_t i = 0; i < points.size(); i++) {
for (size_t j = i+1; j < points.size(); j++) {
double currentDistance = distance(points[i], points[j]);
if (currentDistance < minDistance) {
minDistance = currentDistance;
closestPair.first = points[i];
closestPair.second = points[j];
}
}
}
// 返回最近的点对
std::cout << "Closest pair with distance: " << minDistance << std::endl;
std::cout << "Points: (" << closestPair.first.x << ", " << closestPair.first.y << ", " << closestPair.first.z << ") and (" << closestPair.second.x << ", " << closestPair.second.y << ", " << closestPair.second.z << ")" << std::endl;
}
阅读全文
相关推荐
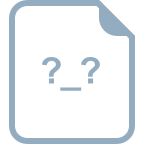
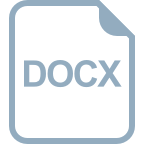
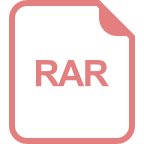
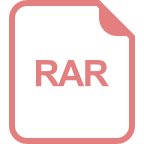
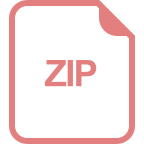
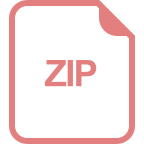
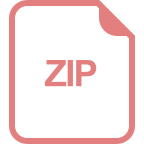
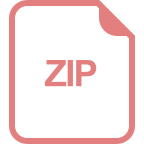
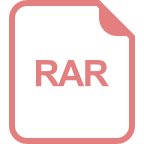
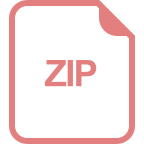
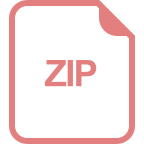
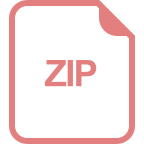
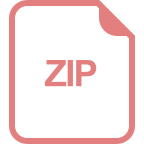
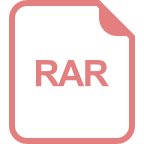
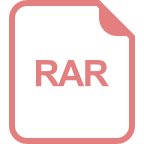
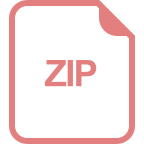