C++用类实现一个学生信息管理系统
时间: 2024-10-17 12:15:45 浏览: 28
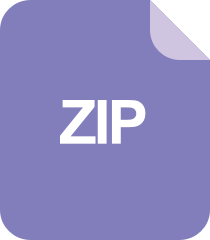
QT C++实现的学生信息管理系统.zip
在C++中,我们可以使用类来实现一个简单的学生成绩管理系统的例子。首先,我们创建一个名为`Student`的基本类,它包含学生的属性如姓名、ID和成绩等。然后,可以添加一些操作,比如设置和获取这些信息的方法:
```cpp
class Student {
public:
// 构造函数
Student(string name, int id)
: name(name), id(id) {}
// 属性
string getName() const { return name; }
int getId() const { return id; }
void setName(string new_name) { name = new_name; }
void setId(int new_id) { id = new_id; }
// 成绩相关方法
double getScore() const { return score; } // 默认假设分数存在
void setScore(double new_score) { score = new_score; }
private:
string name;
int id;
double score;
};
```
接下来,为了支持批量操作,我们可以创建一个`GradeBook`类,作为学生的容器,并提供一些管理功能,例如添加学生、删除学生以及查询平均分等:
```cpp
class GradeBook {
public:
void addStudent(Student& student);
void removeStudent(int id);
void printAverageScore();
private:
map<int, Student> students; // 使用关联数组存储学生
};
// 实现方法...
void GradeBook::addStudent(Student& student) {
students[student.getId()] = student;
}
void GradeBook::removeStudent(int id) {
students.erase(id);
}
void GradeBook::printAverageScore() {
double total_score = 0;
for (const auto& pair : students) {
total_score += pair.second.getScore();
}
if (!students.empty()) {
cout << "Average score: " << total_score / students.size() << endl;
} else {
cout << "No students in the grade book." << endl;
}
}
```
阅读全文
相关推荐
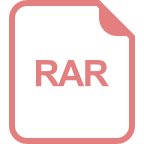
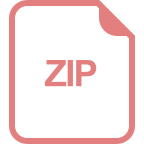
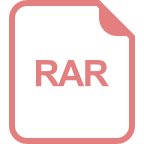
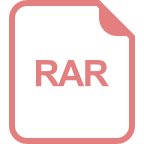
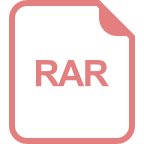
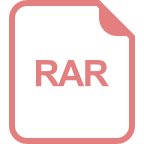
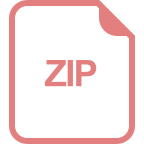
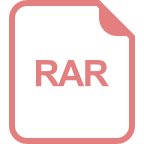
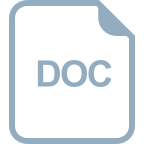
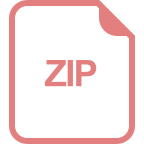
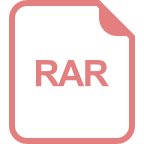
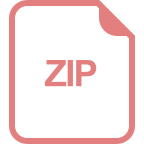
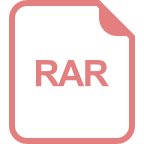
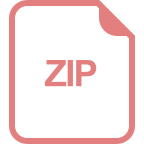
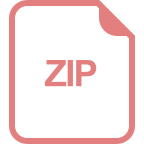
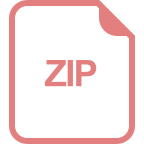
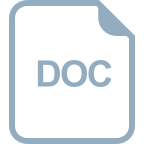