写出使用C++编程语言创建一个文件,通过面向文件的输入输出流(fstream)进行操作,如写入和读取数据,以及如何有效地结合使用标准流对象来实现文件的增删操作的代码?
时间: 2024-10-24 20:03:20 浏览: 12
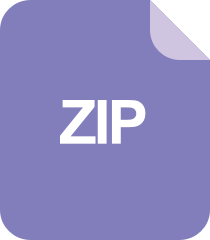
文件读写入链表.zip_C++链表_数据结构_文件读写_读链表_链表读取文本

在C++中,我们可以使用`fstream`库来进行文件操作。下面是一个简单的示例,展示如何创建、写入、读取和删除文件:
```cpp
#include <fstream>
#include <iostream>
// 创建文件并写入数据
void writeToFile(const std::string& filename, const std::string& content) {
std::ofstream file(filename);
if (file.is_open()) {
file << content;
file.close();
std::cout << "Data written to file successfully." << std::endl;
} else {
std::cerr << "Unable to open the file for writing." << std::endl;
}
}
// 从文件中读取数据
std::string readFromFile(const std::string& filename) {
std::ifstream inputFile(filename);
if (inputFile.is_open()) {
std::string line;
getline(inputFile, line); // 一次读一行
inputFile.close();
return line; // 返回读取的内容
} else {
std::cerr << "Unable to open the file for reading." << std::endl;
return "";
}
}
// 删除文件
bool deleteFile(const std::string& filename) {
if (std::remove(filename.c_str()) == 0) {
std::cout << "File deleted successfully." << std::endl;
return true;
} else {
std::cerr << "Failed to delete the file." << std::endl;
return false;
}
}
int main() {
std::string filename = "example.txt";
// 写入文件
writeToFile(filename, "Hello, World!");
// 读取文件内容
std::string content = readFromFile(filename);
std::cout << "Content of the file: " << content << std::endl;
// 删除文件(注意:此操作不可逆,请谨慎)
bool isDeleted = deleteFile(filename);
if (isDeleted)
std::cout << "Cleaning up...";
return 0;
}
```
阅读全文
相关推荐
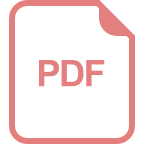
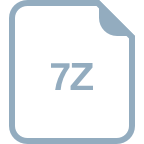
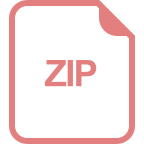
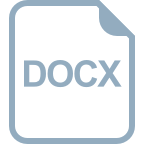
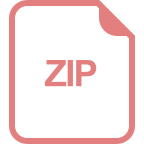
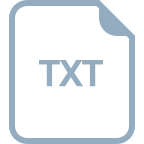
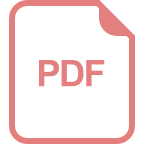
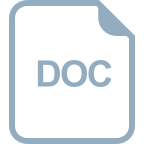
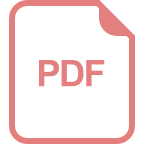
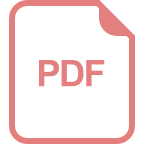
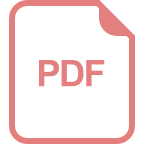
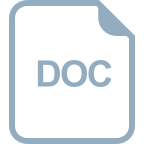
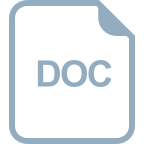
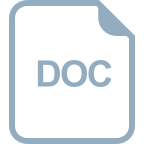
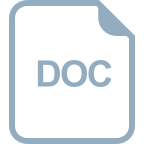
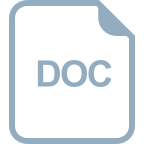
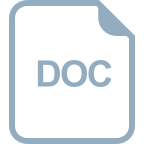
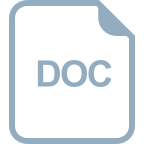