已知92号汽油,每升6元;如果大于等于20升,那么每升5.9 已知97号汽油,每升7元;如果大于等于40升,那么每升6.5 编写JS程序,用户输入自己的汽油编号,然后输入自己加多少升, 在通过使用字符模板在网页上输出相关内容。
时间: 2024-09-27 22:07:44 浏览: 37
为了编写一个JavaScript程序,你可以创建一个函数,该函数接受用户输入的汽油编号和加油量,然后根据提供的价格规则计算总价。这里是一个简单的示例:
```javascript
function calculatePrice(gasolineType, volume) {
let pricePerLiter;
let discountApplied = false;
switch (gasolineType) {
case '92':
pricePerLiter = 6;
if (volume >= 20) {
pricePerLiter = 5.9;
discountApplied = true;
}
break;
case '97':
pricePerLiter = 7;
if (volume >= 40) {
pricePerLiter = 6.5;
discountApplied = true;
}
break;
default:
// 检查输入是否合法
console.error('Invalid gasoline type. Please choose between "92" and "97".');
return;
}
const totalPrice = volume * pricePerLiter;
// 显示结果,包括折扣信息(如果有)
let output = `You purchased ${volume} liters of ${gasolineType} gasoline at ${pricePerLiter} per liter.`;
if (discountApplied) {
output += ` A discount of 0.1 yuan/liter was applied due to the purchase volume being greater than or equal to ${discountVolume}.`;
}
output += ` The total cost is ${totalPrice.toFixed(2)} yuan.`;
return output;
}
// 示例用法
const gasolineTypeInput = prompt("Enter your gasoline type (92 or 97):");
const volumeInput = parseInt(prompt("Enter the volume in liters:"));
if (isNaN(volumeInput)) {
console.error('Invalid volume. Please enter a number.');
} else {
const result = calculatePrice(gasolineTypeInput, volumeInput);
document.getElementById('output').innerText = result;
}
```
在这个代码片段中,我们首先检查用户输入的汽油类型和加油量,然后根据条件设置价格。最后,我们在网页上显示结果。如果用户输入的体积满足折扣条件,还会额外展示折扣信息。
将此代码粘贴到HTML文件中,并确保有一个id为`output`的元素来显示结果。例如:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Gasoline Price Calculator</title>
</head>
<body>
<h2>Gasoline Price Calculation:</h2>
<p id="output"></p>
... (剩下的HTML代码)
<script src="gasoline-calculator.js"></script>
</body>
</html>
```
运行这个程序,用户可以在浏览器中输入汽油类型和加油量,然后看到相应的计算结果。
阅读全文
相关推荐
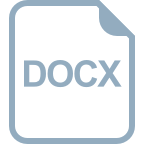
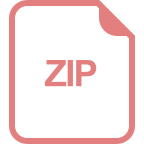
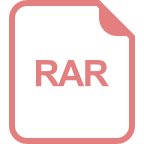

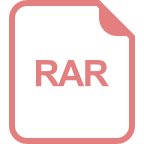
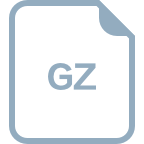
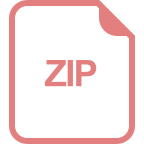
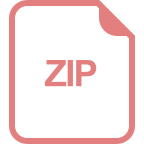
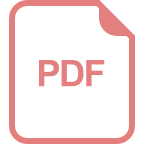
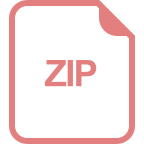
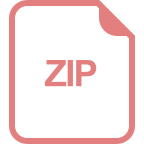
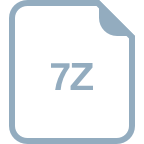
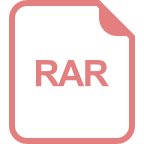
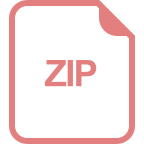
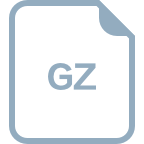
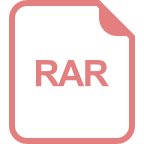
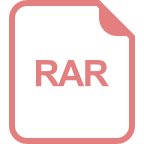
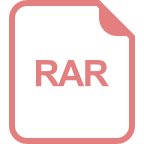
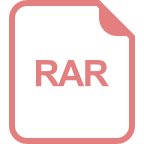