js let变量划线
时间: 2024-09-12 13:09:31 浏览: 29
在JavaScript中,`let` 关键字是ES6(ECMAScript 2015)引入的,用于声明一个块作用域的局部变量。使用 `let` 声明的变量只能在其声明的块或子块中访问,这称为块级作用域。与之相对的是 `var` 关键字,它声明的变量具有函数作用域或全局作用域。`let` 关键字的引入为JavaScript带来了更多的变量作用域控制,有助于避免变量提升(hoisting)和闭包相关的问题。
下面是一个使用 `let` 关键字声明变量的简单例子:
```javascript
if (true) {
let a = 10;
console.log(a); // 输出:10
}
// 下面这行代码会抛出 ReferenceError,因为a的作用域仅限于上面的if块内
console.log(a); // ReferenceError: a is not defined
```
由于 `let` 声明的变量具有块级作用域,因此当使用 `let` 关键字声明变量时,它们只能在声明它们的块内被访问,从而避免了 `var` 可能引起的变量提升问题和全局作用域污染。
`let` 声明的变量可以被重新赋值,但是不能在同一作用域内重复声明,尝试这样做将会抛出一个语法错误(SyntaxError)。这与 `const` 关键字不同,`const` 用于声明一个块作用域的常量,声明后不允许重新赋值。
相关问题
let ctx = uni.createCanvasContext('cpbg', this);
在uni-app框架中,`uni.createCanvasContext()` 是一个用于创建 Canvas 绘画上下文的方法。这里 `ctx` 是一个变量名,它代表一个 CanvasRenderingContext2D 对象,这个对象允许你在 webview 或者 canvas 元素上进行图形绘制。
`'cpbg'` 可能是一个特定的canvas容器id或者是组件的一个属性名称,表示你想在这个特定的canvas元素 'cpbg' 上进行操作,而 `this` 指代的是当前调用该函数的对象,通常会关联到某个vue组件实例。
例如,你可以使用这个上下文来绘制图形、添加文本、设置样式等。下面是一些基本用法:
```javascript
// 创建并初始化canvas context
let ctx = uni.createCanvasContext('cpbg', this);
// 设置线条颜色
ctx.strokeStyle = '#000';
// 绘制一条线
ctx.beginPath();
ctx.moveTo(10, 10);
ctx.lineTo(50, 50);
ctx.stroke();
```
帮我用js画布画一个象棋棋盘
好的,以下是一个简单的实现:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>象棋棋盘</title>
<style>
canvas {
border: 1px solid #000;
}
</style>
</head>
<body>
<canvas id="chessboard"></canvas>
<script>
const canvas = document.getElementById('chessboard');
const ctx = canvas.getContext('2d');
const width = canvas.width = 500;
const height = canvas.height = 500;
const row = 10;
const col = 9;
const gridWidth = width / (col + 1);
const gridHeight = height / (row + 1);
const xStart = gridWidth;
const yStart = gridHeight;
// 绘制棋盘格子
function drawGrid() {
for (let i = 0; i < col; i++) {
for (let j = 0; j < row; j++) {
const x = xStart + i * gridWidth;
const y = yStart + j * gridHeight;
if ((i + j) % 2 === 0) {
ctx.fillStyle = '#b58863';
} else {
ctx.fillStyle = '#f0d9b5';
}
ctx.fillRect(x, y, gridWidth, gridHeight);
}
}
}
// 绘制棋盘边框和线条
function drawBorder() {
ctx.strokeStyle = '#000';
ctx.lineWidth = 2;
ctx.strokeRect(xStart, yStart, col * gridWidth, row * gridHeight);
for (let i = 1; i < col; i++) {
ctx.beginPath();
ctx.moveTo(xStart + i * gridWidth, yStart);
ctx.lineTo(xStart + i * gridWidth, yStart + 4 * gridHeight);
ctx.moveTo(xStart + i * gridWidth, yStart + 5 * gridHeight);
ctx.lineTo(xStart + i * gridWidth, yStart + row * gridHeight);
ctx.stroke();
}
for (let i = 1; i < row - 1; i++) {
ctx.beginPath();
ctx.moveTo(xStart, yStart + i * gridHeight);
ctx.lineTo(xStart + 2 * gridWidth, yStart + i * gridHeight);
ctx.moveTo(xStart + 7 * gridWidth, yStart + i * gridHeight);
ctx.lineTo(xStart + col * gridWidth, yStart + i * gridHeight);
ctx.stroke();
}
}
drawGrid();
drawBorder();
</script>
</body>
</html>
```
这段代码使用了 HTML5 的 `<canvas>` 元素,通过 JavaScript 在画布上绘制了一个象棋棋盘。具体实现过程如下:
1. 使用 `document.getElementById()` 方法获取到 `<canvas>` 元素,并获取到其上下文对象 `ctx`。
2. 定义棋盘的行列数、格子宽度和高度、起始位置等变量。
3. 编写 `drawGrid()` 函数,使用双重循环绘制棋盘格子。对于每个格子,根据奇偶性选择不同颜色填充。
4. 编写 `drawBorder()` 函数,绘制棋盘边框和线条。使用 `strokeRect()` 方法绘制矩形边框,使用 `beginPath()`、`moveTo()` 和 `lineTo()` 方法绘制线条。注意水平线条只需绘制一半,因为棋盘是对称的。
5. 调用 `drawGrid()` 和 `drawBorder()` 函数以绘制完成的棋盘。
阅读全文
相关推荐
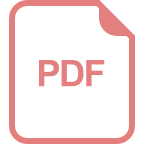
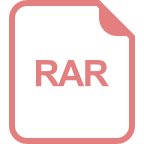
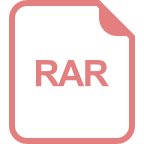
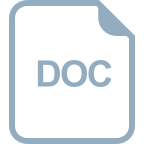
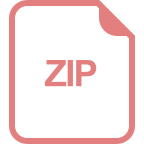
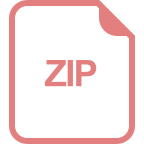
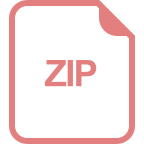
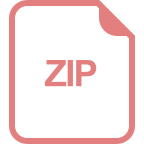
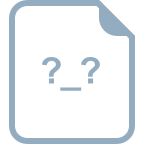
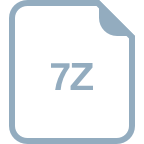
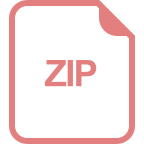
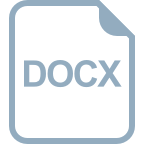
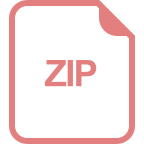
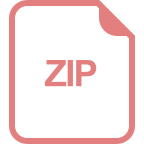
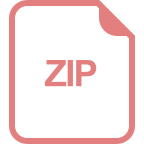
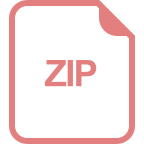
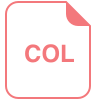
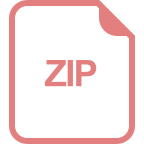