asp mvc 用脚本弹出消息框
时间: 2024-10-18 17:09:23 浏览: 17
在ASP.NET MVC中,如果你想通过JavaScript脚本来显示一个消息提示框,通常会使用客户端的JavaScript库,如jQuery、Vue.js或原生JavaScript。这里以简单的jQuery为例:
```javascript
$(document).ready(function() {
// 当某个操作完成后,比如表单提交成功
$.ajax({
type: 'POST',
url: '@Url.Action("YourActionName", "YourControllerName")', // 替换为你的实际控制器动作
success: function(result) {
// 如果操作成功,显示一个信息框
alert('操作完成,结果是:' + result);
},
error: function(xhr, status, error) {
// 处理错误情况
alert('发生错误:' + xhr.responseText);
}
});
});
```
在这个例子中,`YourActionName`是你的控制器里的一个方法,它应该返回一个字符串或者其他可以传递给`alert`的消息。将URL替换为你实际需要调用的API或视图。
相关问题
asp.net mvc弹出框
要在 ASP.NET MVC 中显示弹出框,可以使用 JavaScript 库或插件。以下是一些可供选择的示例:
1.使用 jQuery UI 对话框:
在 View 中引用 jQuery UI 库和样式文件:
```
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/jquery-1.12.4.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
```
然后在 JavaScript 文件中添加以下代码:
```
$(function() {
$( "#dialog" ).dialog({
autoOpen: false,
modal: true
});
$( "#opener" ).click(function() {
$( "#dialog" ).dialog( "open" );
});
});
```
在 HTML 中添加一个按钮,当点击时打开对话框:
```
<button id="opener">Show Dialog</button>
<div id="dialog" title="Dialog Title">
<p>Dialog content goes here.</p>
</div>
```
2.使用 Bootstrap 模态框:
在 View 中引用 Bootstrap 库和样式文件:
```
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
```
然后在 HTML 中添加以下代码:
```
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#myModal">Open Modal</button>
<div id="myModal" class="modal fade" role="dialog">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">×</button>
<h4 class="modal-title">Modal Title</h4>
</div>
<div class="modal-body">
<p>Modal content goes here.</p>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
```
当按钮被点击时,模态框会弹出显示。
C# core web 弹出消息框
在 ASP.NET Core Web 应用程序中,不能直接使用 JavaScript 的 `alert` 方法弹出消息框,因为它涉及到与客户端的交互,而 ASP.NET Core 采用的是基于服务器的代码模型。不过,可以使用 JavaScript 的替代方法,在客户端上弹出消息框。
1. 在 HTML 页面中添加一个按钮,并在该按钮的 `onclick` 事件中调用 JavaScript 方法。
```html
<button onclick="showMessage()">点击查看消息</button>
```
2. 在 JavaScript 中编写 `showMessage` 方法,使用 `console.log` 方法将消息输出到控制台。
```javascript
function showMessage() {
console.log("这是一条消息!");
}
```
3. 在 C# 中,可以使用 `IJSRuntime` 接口在客户端上执行 JavaScript 代码,从而在 Web 页面中弹出消息框。
```csharp
using Microsoft.AspNetCore.Mvc;
using Microsoft.JSInterop;
using System.Threading.Tasks;
public class HomeController : Controller
{
private readonly IJSRuntime _jsRuntime;
public HomeController(IJSRuntime jsRuntime)
{
_jsRuntime = jsRuntime;
}
public async Task<IActionResult> Index()
{
string script = "showMessage()";
await _jsRuntime.InvokeVoidAsync("eval", script);
return View();
}
}
```
在 `Index` 方法中,使用 `IJSRuntime` 接口调用 `eval` 方法执行 JavaScript 代码,从而在客户端上弹出消息框。
当用户单击按钮时,将会在客户端上弹出一个消息框,显示文本为“这是一条消息!”。
阅读全文
相关推荐
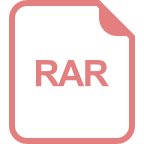
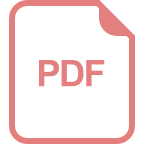
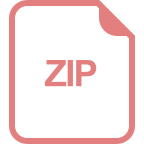













