问题描述 编写一个函数,接收一个字符串作为输入,并统计并输出字符串中每个单词出现的次数。 输入描述 用户输入一个字符串。 输出描述 输出一个字典,键是单词,值是该单词出现的次数。
时间: 2024-10-17 22:12:37 浏览: 53
要编写一个函数来统计给定字符串中每个单词出现的次数,你可以使用Python的内置数据结构`collections.Counter`,这个工具非常适合计数元素。这里是一个简单的示例:
```python
from collections import Counter
def word_count(input_string):
# 首先,我们需要清理输入字符串,移除标点符号并将所有字母转换为小写
cleaned_string = input_string.lower().replace(',', '').replace('.', '').replace('!', '').replace('?', '').split()
# 使用Counter计算单词频率
word_counts = Counter(cleaned_string)
return word_counts
# 示例用法
input_text = "Hello, world! This is a test. Hello again."
word_frequency = word_count(input_text)
print(word_frequency)
```
在这个例子中,我们首先将输入字符串中的标点符号替换为空格,然后分割成单词列表。接着,我们将单词列表传递给`Counter`函数,它会返回一个字典,其中的键是单词,值是该单词出现的次数。
相关问题
用python编写一个函数,接收一个字符串作为输入,并统计并输出字符串中每个单词出现的次数(输出一个字典,键是单词,值是该单词出现的次数。)。不使用python内置数据结构。
在Python中,我们可以编写一个函数来统计字符串中每个单词的出现次数,不过为了不使用内置数据结构,我们可以使用列表和简单的遍历来完成这个任务。下面是一个示例函数:
```python
def word_count(s):
# 将字符串转换为小写并分割成单词列表
words = s.lower().split()
# 创建一个空列表来存储计数
word_counts = [0] * len(words)
# 遍历单词列表
for i in range(len(words)):
# 更新对应位置的计数
word_counts[i] += 1
# 创建一个字典,将索引映射回原始单词
word_dict = {words[i]: counts for i, counts in enumerate(word_counts)}
return word_dict
# 示例使用
input_string = "Hello world! This is a test string."
result = word_count(input_string)
print(result)
```
使用Java,编写程序,接收一个字符串,实现字符串中每个单词的首字母改为大写并输出。
可以使用Java的字符串操作函数和循环语句实现该功能。具体代码如下:
```java
import java.util.Scanner;
public class CapitalizeFirstLetter {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("请输入一个字符串:");
String str = input.nextLine();
String[] words = str.split(" ");
String result = "";
for (int i = 0; i < words.length; i++) {
String word = words[i];
String firstLetter = word.substring(0, 1).toUpperCase();
String restLetters = word.substring(1);
result += firstLetter + restLetters + " ";
}
System.out.println("每个单词首字母大写后的字符串为:" + result.trim());
}
}
```
代码执行流程:
1. 首先使用Scanner类获取用户输入的字符串。
2. 然后使用split函数将字符串按照空格分割成单词。
3. 循环遍历每个单词,将其首字母改为大写,并将结果拼接到一个新的字符串中。
4. 最后输出新的字符串,注意要去除末尾的空格。
阅读全文
相关推荐
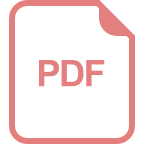
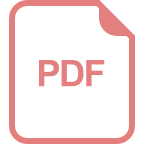
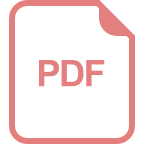













