用Python写 输入一个非空字符串,去除重复的字符后,从小到大排序,输出为一个新字符串
时间: 2024-11-05 15:27:10 浏览: 42
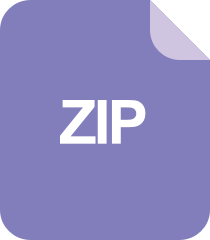
人工智能-项目实践-python-python lis列表排序,按字符串长度从小到大排序.zip
在 Python 中,你可以通过以下步骤实现这个功能:
1. 首先,接收用户输入的非空字符串。
2. 使用 `set` 数据结构来去除重复的字符,因为集合内的元素都是唯一的。
3. 将集合转换回列表,因为集合是无序的,需要转化为列表才能排序。
4. 对列表进行排序,由于默认的排序是字典顺序(ASCII码),所以可以直接使用 `sorted()` 函数。
5. 最后,将排序后的列表连接成一个新的字符串。
下面是一个示例函数来完成这个任务:
```python
def remove_duplicates_sort_string(input_str):
unique_chars = list(set(input_str)) # 去除重复并转为列表
sorted_chars = ''.join(sorted(unique_chars)) # 排序并将字符连接成新的字符串
return sorted_chars
# 示例
input_string = input("请输入一个非空字符串:")
result = remove_duplicates_sort_string(input_string)
print(f"去重并排序后的结果是:{result}")
阅读全文
相关推荐
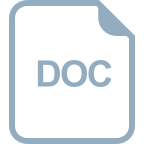
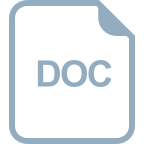











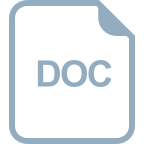
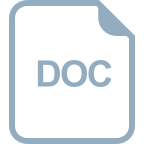
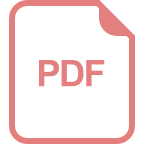
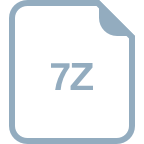