输入年和月,输出"XX年XX月有XX天" 需要考虑闰年的2月有29天 闰年:可以被4整除并且不可以被100整除,或者可以被400整除
时间: 2024-10-13 13:10:21 浏览: 22
对于这个问题,你需要编写一个程序来计算给定年份和月份有多少天。对于非二月,一般月份都有固定的天数(如1月有31天,3月有31天等),而对于二月,需要特殊处理闰年的情况。下面是一个基本的Python函数示例:
```python
def days_in_month(year, month):
months_days = {
1: 31,
2: 29 if is_leap_year(year) else 28,
3: 31,
4: 30,
5: 31,
6: 30,
7: 31,
8: 31,
9: 30,
10: 31,
11: 30,
12: 31,
}
return f"{year}年{month}月有{months_days[month]}天"
def is_leap_year(year):
return (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0)
# 测试
print(days_in_month(2020, 2)) # 输出:2020年2月有29天
```
相关问题
闰年规则:能被4整除,并且不能被100整除,或者能被400整除的年份称之为闰年。 编写程序,用户输入年份,输出该年份是闰年或者不是闰年。例如: 输入: 请输入年份:1900 输出: 1900年不是闰年
根据题意,我们只需要判断用户输入的年份是否符合闰年的规则即可。具体实现如下(以 Python 为例):
```python
year = int(input("请输入年份:"))
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
print(year, "年是闰年")
else:
print(year, "年不是闰年")
```
我们可以输入样例中的数据进行测试:
```
请输入年份:1900
1900 年不是闰年
```
可以看到,1900 年不符合闰年规则,输出结果为“1900 年不是闰年”。
国年规则:能被4整除,并且不能被100整除,或者能被400整除的年份称之为国年。 编写程序,用户输入年份,输出该年份是国年或者不是闰年。例如: 输入: 请输入年份:1900 输出: 1900年不是闰年
好的,我知道了。以下是 Python 代码实现:
```
year = int(input("请输入年份:"))
if year % 4 == 0 and year % 100 != 0 or year % 400 == 0:
print(year, "年是闰年")
else:
print(year, "年不是闰年")
```
如果输入的年份能被4整除并且不能被100整除,或者能被400整除,就是国年,也就是闰年。否则就不是闰年。
阅读全文
相关推荐
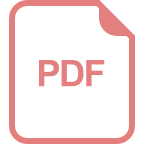
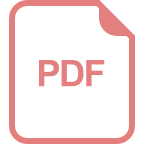
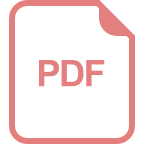












