MVVM用到哪些编程语法技术,C#编程写一个简单的MVVM例子
时间: 2024-12-15 12:25:33 浏览: 15
MVVM(Model-View-ViewModel)是一种架构模式,常用于WPF(Windows Presentation Foundation)和UWP(Universal Windows Platform)等框架的用户界面设计中。它主要使用了以下几个编程技术:
1. **依赖注入**:用于管理应用程序中的组件和服务之间的松耦合,比如通过构造函数、属性注入等方式将ViewModel与View分离。
2. **观察者模式**:ViewModel通过发布通知(例如INotifyPropertyChanged),当数据模型变化时,通知视图自动更新。
3. **命令系统**:利用ICommand接口处理用户操作,使得逻辑独立于UI,提高代码可测试性和可维护性。
4. **数据绑定**:如XAML中的DataBinding特性,可以直接将ViewModel的数据属性绑定到UI元素上,简化UI更新过程。
下面是一个简单的C# MVVM在XAML和C#代码中的示例:
```xml (XAML)
<!-- View (Window.xaml) -->
<Window x:Class="MVVMExample.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<StackPanel>
<TextBox Text="{Binding MyText, Mode=TwoWay}" />
<Button Content="Change Text" Command="{Binding ChangeTextCommand}" />
</StackPanel>
</Window>
```
```csharp (C#)
// ViewModel (MainWindowViewModel.cs)
using System.ComponentModel;
public class MainWindowViewModel : INotifyPropertyChanged
{
private string myText;
public string MyText
{
get => myText;
set
{
if (value != myText)
{
myText = value;
OnPropertyChanged("MyText");
}
}
}
private ICommand changeTextCommand;
public ICommand ChangeTextCommand => new RelayCommand(() => MyText += " Changed!");
// Implement INotifyPropertyChanged
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged([CallerMemberName] string propertyName = null)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
// Command (use a library like GalaSoft.MvvmLight for this)
using GalaSoft.MvvmLight.Command;
public class RelayCommand : ICommand
{
// ...
}
```
在这个例子中,`MainWindowViewModel`是ViewModel,`TextBox`是View,`MyText`属性用于双向数据绑定,并且`ChangeTextCommand`是命令,当按钮点击时会改变文本并触发视图更新。
阅读全文
相关推荐









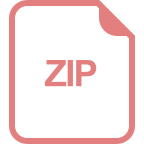






