Linux 四个线程发送一个线程回复消息对应的四个线程
时间: 2023-11-28 21:45:01 浏览: 83
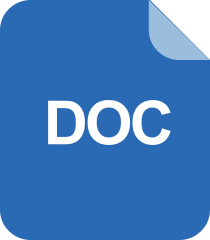
linux下多线程编程

您好!对于 Linux 中四个线程发送消息并回的场景,我们可以使用线程间的消息队列进行通信。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <unistd.h>
#define MAX_MSG_LEN 100
typedef struct {
int sender_id;
char message[MAX_MSG_LEN];
} Message;
pthread_mutex_t mutex;
pthread_cond_t cond;
Message msg_queue[4];
int msg_count = 0;
void* thread_function(void* arg) {
int thread_id = *(int*)arg;
int receiver_id = (thread_id + 1) % 4; // 接收者线程ID
while (1) {
pthread_mutex_lock(&mutex);
// 检查是否有消息
while (msg_count == 0 || msg_queue[receiver_id].sender_id != thread_id) {
pthread_cond_wait(&cond, &mutex);
}
// 处理消息
printf("Thread %d received message: %s\n", receiver_id, msg_queue[receiver_id].message);
// 更新消息计数
msg_count--;
msg_queue[receiver_id].sender_id = -1;
pthread_mutex_unlock(&mutex);
pthread_cond_signal(&cond);
sleep(1); // 等待一段时间继续接收消息
}
}
int main() {
pthread_t threads[4];
int thread_ids[4] = {0, 1, 2, 3};
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
for (int i = 0; i < 4; i++) {
pthread_create(&threads[i], NULL, thread_function, &thread_ids[i]);
}
// 主线程发送消息
int sender_id = 0;
while (1) {
pthread_mutex_lock(&mutex);
// 检查是否有空闲的消息槽
while (msg_count >= 4 || msg_queue[sender_id].sender_id != -1) {
pthread_cond_wait(&cond, &mutex);
}
// 发送消息
snprintf(msg_queue[sender_id].message, MAX_MSG_LEN, "Message from thread %d", sender_id);
msg_queue[sender_id].sender_id = sender_id;
msg_count++;
pthread_mutex_unlock(&mutex);
pthread_cond_signal(&cond);
sender_id = (sender_id + 1) % 4; // 切换到下一个发送者线程
sleep(1); // 等待一段时间继续发送消息
}
return 0;
}
```
这个示例代码创建了四个线程,它们通过共享的消息队列进行通信。每个线程负责接收来自其他线程的消息,并输出接收到的消息。主线程负责发送消息,每个线程按照顺序发送消息给下一个线程。
希望这个示例能够帮助您理解如何在 Linux 中实现四个线程之间的消息发送和回复。如果有任何问题,请随时提问!
阅读全文
相关推荐
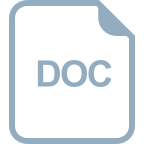
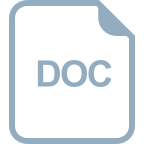
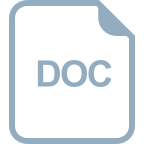
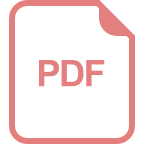
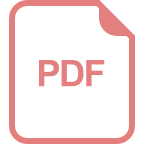
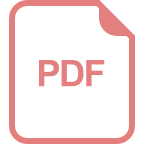
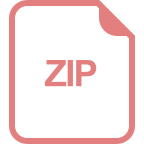
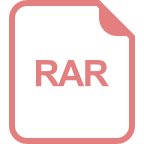
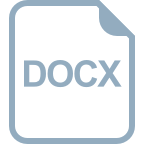
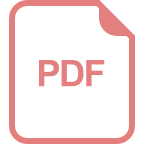
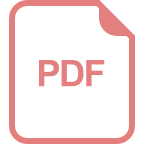
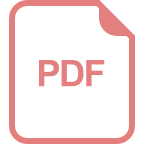
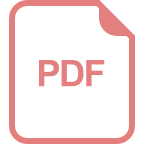
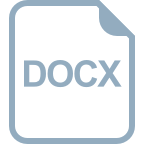
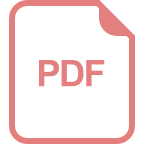
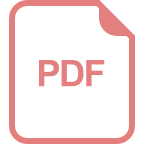
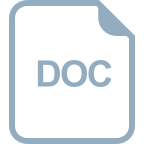
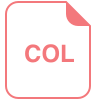