写一个HTML时钟代码,要求有表盘,时针,分针,秒针
时间: 2024-09-28 20:15:21 浏览: 36
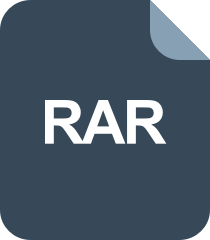
HTML5带表盘和数字刻度时钟代码
要创建一个HTML时钟,你可以使用CSS和JavaScript来实现基本的功能。以下是一个简单的例子,包括一个带有数字的圆形表盘、时针、分针和秒针:
```html
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<style>
#clock {
position: relative;
width: 200px;
height: 200px;
background-color: #f5f5f5;
border-radius: 50%;
display: flex;
justify-content: center;
align-items: center;
}
.hand {
position: absolute;
width: 6px;
height: 60px;
transform-origin: bottom center;
}
.hour-hand, .minute-hand, .second-hand {
animation-duration: 1s, 60s, 1000ms;
animation-timing-function: steps(12, end);
}
</style>
</head>
<body>
<div id="clock">
<!-- 表盘 -->
<div class="face">
<svg viewBox="0 0 200 200" style="display: none;">
<!-- 添加12个小时的数字 -->
<text x="100%" y="50%" dominant-baseline="middle" text-anchor="middle" font-size="24">
<tspan>12</tspan><tspan dy="20">1</tspan><tspan dy="20">2</tspan><tspan dy="20">3</tspan>
...
<tspan dy="20">9</tspan><tspan dy="20">10</tspan><tspan dy="20">11</tspan>
</text>
</svg>
</div>
<!-- 时针 -->
<div class="hand hour-hand"></div>
<!-- 分针 -->
<div class="hand minute-hand"></div>
<!-- 秒针 -->
<div class="hand second-hand"></div>
</div>
<script>
function updateHands() {
var now = new Date();
var hours = now.getHours();
var minutes = now.getMinutes();
var seconds = now.getSeconds();
// 将小时、分钟调整到0 - 11之间(12小时制)
hours %= 12;
if (hours == 0) hours = 12;
// 计算角度
var hourRotation = (hours + minutes / 60 + seconds / 3600) * Math.PI * 2;
var minuteRotation = minutes * Math.PI * 2 / 30;
var secondRotation = seconds * Math.PI * 2 / 60;
// 更新手的位置
document.querySelector('.hour-hand').style.transform = `rotate(${hourRotation}rad)`;
document.querySelector('.minute-hand').style.transform = `rotate(${minuteRotation}rad)`;
document.querySelector('.second-hand').style.transform = `rotate(${secondRotation}rad)`;
}
setInterval(updateHands, 1000); // 每秒更新一次
</script>
</body>
</html>
```
这个示例中,CSS负责创建一个圆形表盘并定义时针、分针和秒针的基本样式。JavaScript则用于获取当前时间并计算它们在圆周上的位置,然后更新DOM元素的`transform`属性。
阅读全文
相关推荐
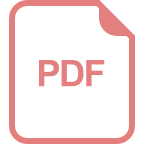
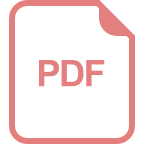


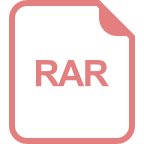
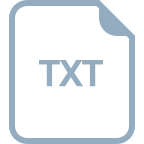
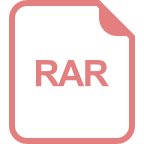
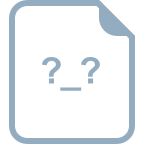
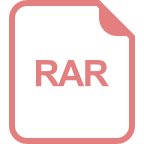
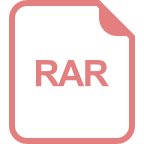
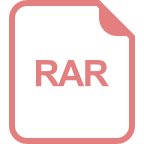
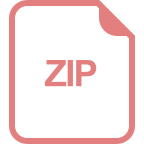
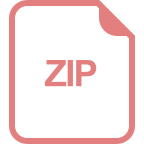




