{ "code": 200, "msg": "操作成功", "errorField": null, "timestamp": 1687229625922, "data": [ { "id": 1, "name": "Test Station", "longitude": "123.456", "latitude": "78.910", "province": "Test Province", "city": "Test City", "county": "Test County", "address": "Test Address", "installedPower": "1000W", "capacity": "1000J", "operationTime": "2023-6-23", "emsType": 0 }, { "id": 1, "name": "Power Station 1", "longitude": "123.456", "latitude": "78.90", "province": "Province 1", "city": "City 1", "county": "County 1", "address": "Address 1", "installedPower": "1000W", "capacity": "1000J", "operationTime": "2022-01-01", "emsType": 0 } ] }这是响应成功后的内容,如何拿到data里的值
时间: 2023-07-24 13:14:44 浏览: 114
要获取响应成功后"data"字段中的值,你可以在代码中进行解析和处理。根据你提供的响应成功的示例,可以使用JSON解析库(如JSON.parseObject)来解析JSON字符串,并获取"data"字段的值。
以下是一个示例代码片段,演示如何从响应成功的内容中获取"data"字段的值:
```java
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
// ...
// 假设响应成功的内容为responseJson字符串
String responseJson = "{ \"code\": 200, \"msg\": \"操作成功\", \"errorField\": null, \"timestamp\": 1687229625922, \"data\": [ { \"id\": 1, \"name\": \"Test Station\", \"longitude\": \"123.456\", \"latitude\": \"78.910\", \"province\": \"Test Province\", \"city\": \"Test City\", \"county\": \"Test County\", \"address\": \"Test Address\", \"installedPower\": \"1000W\", \"capacity\": \"1000J\", \"operationTime\": \"2023-6-23\", \"emsType\": 0 }, { \"id\": 1, \"name\": \"Power Station 1\", \"longitude\": \"123.456\", \"latitude\": \"78.90\", \"province\": \"Province 1\", \"city\": \"City 1\", \"county\": \"County 1\", \"address\": \"Address 1\", \"installedPower\": \"1000W\", \"capacity\": \"1000J\", \"operationTime\": \"2022-01-01\", \"emsType\": 0 } ] }";
JSONObject jsonObject = JSON.parseObject(responseJson);
JSONArray data = jsonObject.getJSONArray("data");
// 遍历"data"数组,获取每个元素的值
for (int i = 0; i < data.size(); i++) {
JSONObject station = data.getJSONObject(i);
int id = station.getIntValue("id");
String name = station.getString("name");
String longitude = station.getString("longitude");
String latitude = station.getString("latitude");
// 其他字段的获取
// ...
// 打印每个站点的信息
System.out.println("Station ID: " + id);
System.out.println("Station Name: " + name);
System.out.println("Station Longitude: " + longitude);
System.out.println("Station Latitude: " + latitude);
// 其他字段的输出
// ...
}
```
在上述代码中,首先使用JSON.parseObject方法将响应成功的内容转换为JSON对象。然后通过getJSONArray方法获取"data"字段对应的数组。接下来,可以使用循环遍历数组,通过getJSONObject方法获取每个站点的信息。根据具体的字段名,使用getIntValue、getString等方法获取字段的值,并进行相应的处理或输出。
注意,上述代码中使用了阿里巴巴的fastjson库来进行JSON解析。你需要确保已将该库导入到项目中,并根据实际情况修改代码中的数据类型和字段名称。
阅读全文
相关推荐
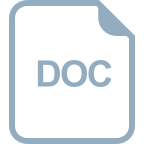
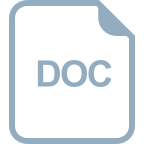
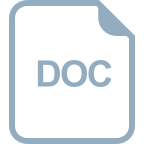
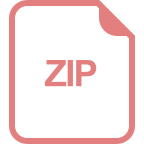
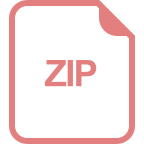
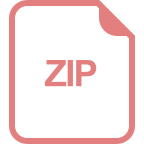
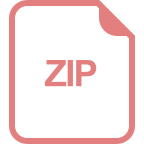
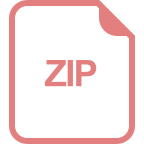
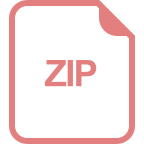
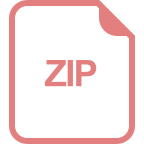
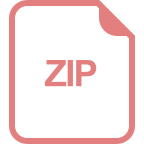
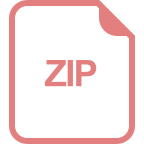
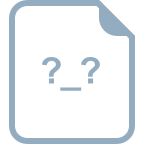
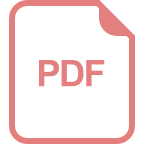
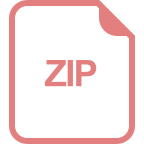
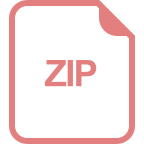
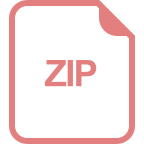
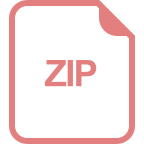
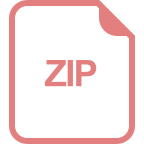