在C语言中,如何使用if语句来检查一个字符是否为字母?
时间: 2024-11-20 20:41:15 浏览: 4
在C语言中,你可以使用ASCII码来判断一个字符是否为字母。ASCII码规定了字符的标准值,其中大写字母'A'到'Z'的ASCII码范围是从65到90,小写字母'a'到'z'的ASCII码范围是从97到122。因此,你可以通过比较一个字符的ASCII值来确定它是否在这个范围内。
以下是检查一个字符是否为字母的基本if语句示例:
```c
#include <ctype.h> // 引入ctype库,包含了isalpha函数
char ch; // 存储需要检查的字符
// 获取用户输入或其他来源的字符
scanf("Input a character: ", &ch);
// 使用if语句检查字符是否为字母
if(isalpha(ch)) {
printf("%c is an alphabet.\n", ch);
} else {
printf("%c is not an alphabet.\n", ch);
}
```
这里的`isalpha()`函数就是从ctype库中获取的,如果字符是字母(包括大写字母和小写字母),它会返回非零值;如果不是,则返回0。所以通过这个函数的判断,我们就可以轻松地知道字符是否为字母了。
相关问题
编写一个C语言程序,如何使用if语句统计输入字符串中英文字母、空格、数字和其它字符的具体数量?
在C语言中,编写一个程序来统计输入字符串中各个字符类型的数量,可以使用`if...else if...else`结构配合`isalpha()`、`isspace()`、`isdigit()`函数以及一些基本计数操作。以下是一个简单的示例:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char input[100];
int alpha_count = 0, space_count = 0, digit_count = 0, other_count = 0;
printf("Enter a string: ");
fgets(input, sizeof(input), stdin); // Read input with newline character
for (int i = 0; input[i] != '\0'; i++) { // Iterate through the string
if (isalpha(input[i])) { // Check if character is an alphabet
alpha_count++;
} else if (isspace(input[i])) { // Check if character is a space
space_count++;
} else if (isdigit(input[i])) { // Check if character is a digit
digit_count++;
} else { // Other characters (including punctuation or special symbols)
other_count++;
}
}
printf("Number of alphabets: %d\n", alpha_count);
printf("Number of spaces: %d\n", space_count);
printf("Number of digits: %d\n", digit_count);
printf("Number of other characters: %d\n", other_count);
return 0;
}
```
在这个程序中,我们首先定义了一个数组来存储用户输入,然后使用`isalpha()`检查是否为字母,`isspace()`检查是否为空格,`isdigit()`检查是否为数字。如果都不是,那么它会被归类到"其他"类别。最后,我们打印出每个类别字符的数量。
c语言编程用if语句实现:输入一个字符,判断它是否为大写字母。如果是,则转换为小写字母输出;若不是,则输出原字符。
在C语言中,你可以使用`if`语句结合`isupper()`函数来实现这个功能。`isupper()`函数用于检查一个字符是否为大写字母。以下是相应的代码:
```c
#include <ctype.h> // 引入ctype库,包含isupper()函数
int main() {
char input;
printf("请输入一个字符: ");
scanf("%c", &input); // 输入字符
if (isupper(input)) { // 判断字符是否为大写字母
input = tolower(input); // 转换为小写字母
printf("转换后的字符是: %c\n", input);
} else {
printf("输入的字符已存在,无需转换: %c\n", input);
}
return 0;
}
```
在这个程序中,如果输入的是大写字母,`isupper()`会返回非零值,`tolower()`将字符转换为小写并打印出来;如果不是大写字母,则直接打印原始字符。
阅读全文
相关推荐
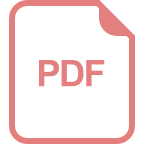
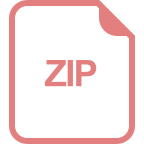
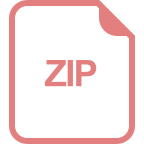













