我是web前端开发工程师,我想实现:在浏览器点击下载按钮,用户选择下载路径,触发下载任务,前提:只有一个下载任务,一次下载多个文件,不能压缩包
时间: 2024-09-13 17:03:00 浏览: 56
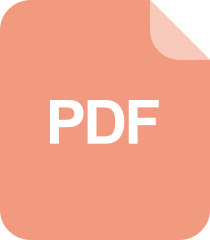
vue实现点击按钮下载文件功能
为了实现在浏览器中单击下载按钮后,允许用户自定义下载路径并一次下载多个文件,但不以压缩包形式,可以采用HTML5的`<a>`元素配合服务器支持的机制。以下是基本步骤:
1. **创建链接**:
- 在HTML中,为每个文件创建一个`<a>`元素,设置`href`属性为每个文件的URL,同时添加`download`属性来指定文件名。
```html
<a id="file1Link" href="/path/to/file1" download="file1.txt">下载文件1</a>
<a id="file2Link" href="/path/to/file2" download="file2.txt">下载文件2</a>
```
2. **事件监听**:
- 给下载按钮添加点击事件,当点击时,获取用户想要下载的文件的链接列表,然后发起下载。
```javascript
const downloadButtons = document.querySelectorAll('.download-button');
downloadButtons.forEach(button => {
button.addEventListener('click', async () => {
const links = Array.from(document.querySelectorAll('#file1Link, #file2Link')); // 获取链接集合
await downloadFiles(links);
});
});
async function downloadFiles(links) {
links.forEach(link => {
link.click();
});
}
```
3. **服务器支持**:
- 在服务器端,确保接收这些请求并返回正确的响应头(例如Content-Disposition),告诉浏览器这是一个下载而不是浏览的内容。
```javascript
// Node.js示例 (Express框架)
app.get('/path/to/download', (req, res) => {
res.setHeader('Content-Type', 'application/octet-stream'); // 设置正确的MIME类型
res.setHeader('Content-Disposition', `attachment; filename="${req.query.filename}"`); // 自定义文件名
res.send(fileData); // 文件数据
});
```
这样,用户点击下载按钮后,浏览器会弹出文件下载框,让用户选择保存位置,然后逐个下载指定的文件。注意,在生产环境中,为了防止跨域问题,可能需要服务器做相应的跨域配置。
阅读全文
相关推荐
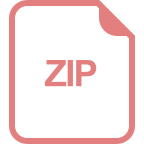
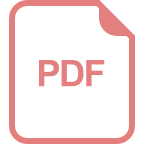
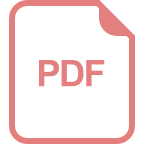
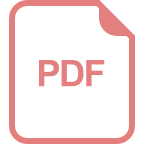
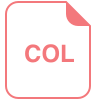
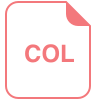
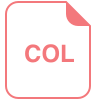
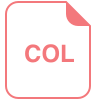
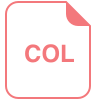
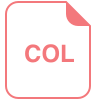
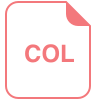
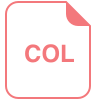
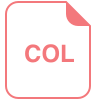
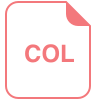
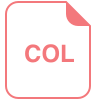
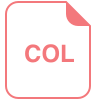
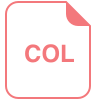
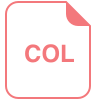