java中每次for循环都生成一个新的Map<String, Object> condition1 = new HashMap<>();将其存放到list中 map的名称不能重复
时间: 2024-10-19 18:11:55 浏览: 19
在Java中,如果你想要在每次for循环中创建一个新的`Map<String, Object>`实例,并将这个新地图放入一个列表中,你可以这样做:
```java
List<Map<String, Object>> conditionMaps = new ArrayList<>();
for (int i = 0; i < someCondition; i++) { // 假设someCondition是你需要迭代的次数
Map<String, Object> condition1 = new HashMap<>(); // 每次循环都会创建一个新的Map对象
// 对condition1添加你需要的数据
condition1.put("key1", value1);
condition1.put("key2", value2);
// 将新的条件地图添加到列表中
conditionMaps.add(condition1);
}
```
在这个例子中,`conditionMaps`是一个存储了多个`HashMap<String, Object>`的列表,它们都是在for循环的不同迭代过程中独立创建的。
相关问题
java中每次for循环都生成一个新的Map<String, Object> condition1 = new HashMap<>(); map的名称不能重复
在Java中,如果你需要在每次for循环中创建新的`Map<String, Object>`实例,确实可以这样做,因为`Map`是引用类型,所以每次分配的是一个新的内存地址。例如:
```java
for (int i = 0; i < someList.size(); i++) {
Map<String, Object> condition1 = new HashMap<>(); // 创建一个新的HashMap实例
// 这里的condition1是一个独立的变量,与其他循环迭代无关
condition1.put("key", "value"); // 对每个新地图添加元素
}
```
然而,如果目的是为了在循环外部能够访问到这些单独的`Map`实例,你可以考虑将它们存储在一个列表或数组中,比如:
```java
List<Map<String, Object>> conditions = new ArrayList<>();
for (int i = 0; i < someList.size(); i++) {
conditions.add(new HashMap<>());
conditions.get(i).put("key", "value");
}
// 现在你可以通过索引来访问每个条件
conditions.get(0); // 获取第一个条件
```
在这种情况下,`conditions`列表会保存每次循环创建的新`Map`。
mybatisPlus Map<String, String>
MyBatis Plus is an enhanced version of the MyBatis framework, which is a popular persistence framework for Java. It simplifies the usage of MyBatis and provides additional features.
In MyBatis Plus, you can use the `Map<String, String>` type to represent key-value pairs. This can be useful in scenarios where you want to pass dynamic parameters to a SQL query or store dynamic properties in a database column.
Here's an example of how you can use `Map<String, String>` with MyBatis Plus:
```java
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.extension.service.additional.query.impl.QueryChainWrapper;
import java.util.HashMap;
import java.util.Map;
public class MyBatisPlusExample {
public static void main(String[] args) {
// Create a map with key-value pairs
Map<String, String> params = new HashMap<>();
params.put("name", "John");
params.put("age", "30");
// Create a query wrapper
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
// Add conditions using the map
params.forEach((key, value) -> queryWrapper.eq(key, value));
// Execute the query
UserMapper userMapper = new UserMapper();
List<User> userList = userMapper.selectList(queryWrapper);
}
}
```
In this example, we create a `Map<String, String>` called `params`, which contains key-value pairs representing the conditions we want to add to the query. We then iterate over the map using `forEach` and add each condition to the `QueryWrapper` using the `eq` method. Finally, we execute the query and retrieve the results.
Note that this is just a basic example, and you can use `Map<String, Object>` if you need to handle values of different types. MyBatis Plus provides many other features and utilities that can simplify your database operations.
阅读全文
相关推荐
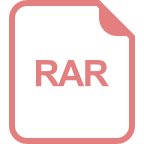
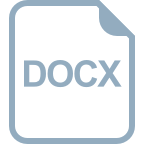
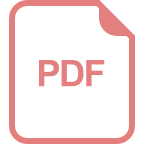
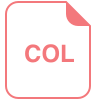
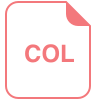
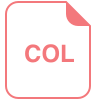
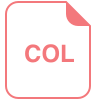
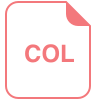
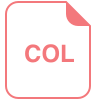
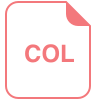
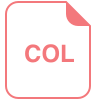
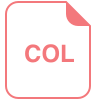
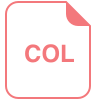



