策略模式实现 if else
时间: 2024-01-30 10:01:28 浏览: 62
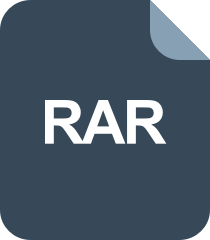
策略模式实现
策略模式是一种行为型设计模式,它可以用来消除繁琐的if-else语句,并实现算法的动态替换。策略模式使用面向对象的继承和多态机制,使得同一行为在不同场景下具备不同的实现。
在策略模式中,我们将不同的算法封装成不同的策略类,每个策略类都实现了一个共同的接口或基类。客户端根据需要选择使用哪个策略类,从而实现不同的行为。
使用策略模式可以避免代码中大量的if-else语句,提高代码的可读性和可维护性。同时,策略模式也符合开闭原则,可以方便地添加新的策略类。
以下是一个使用策略模式实现if-else的示例:
```python
# 定义策略接口
class Strategy:
def do_operation(self):
pass
# 定义具体的策略类
class StrategyA(Strategy):
def do_operation(self):
print("执行策略A")
class StrategyB(Strategy):
def do_operation(self):
print("执行策略B")
class StrategyC(Strategy):
def do_operation(self):
print("执行策略C")
# 定义上下文类
class Context:
def __init__(self, strategy):
self.strategy = strategy
def execute_strategy(self):
self.strategy.do_operation()
# 客户端代码
strategy_a = StrategyA()
strategy_b = StrategyB()
strategy_c = StrategyC()
context = Context(strategy_a)
context.execute_strategy() # 输出:执行策略A
context = Context(strategy_b)
context.execute_strategy() # 输出:执行策略B
context = Context(strategy_c)
context.execute_strategy() # 输出:执行策略C
```
通过使用策略模式,我们可以将不同的行为封装成不同的策略类,客户端根据需要选择使用哪个策略类,从而实现不同的行为。这样就避免了繁琐的if-else语句,使代码更加清晰和可扩展。
阅读全文
相关推荐
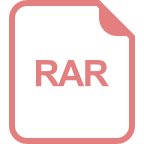

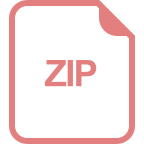
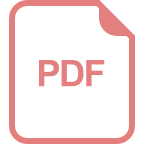
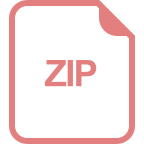
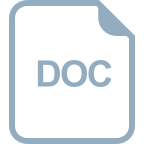










