RestTemplate异常处理
时间: 2023-10-12 08:06:21 浏览: 274
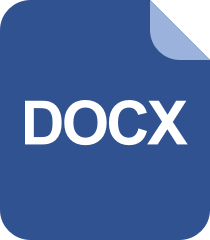
精讲RestTemplate自定义请求失败异常处理.docx
对于使用 RestTemplate 发起 HTTP 请求时可能出现的异常,可以进行以下异常处理:
1. HttpClientErrorException:当响应的状态码为 4xx(如 404 Not Found)时,会抛出此异常。可以通过 `getStatusCode()` 方法获取状态码,`getResponseBodyAsString()` 获取错误响应的内容。
```java
try {
ResponseEntity<String> response = restTemplate.exchange(url, HttpMethod.GET, null, String.class);
// 处理成功响应
} catch (HttpClientErrorException e) {
if (e.getStatusCode() == HttpStatus.NOT_FOUND) {
// 处理 404 错误
} else {
// 处理其他 4xx 错误
}
}
```
2. HttpServerErrorException:当响应的状态码为 5xx(如 500 Internal Server Error)时,会抛出此异常。
```java
try {
ResponseEntity<String> response = restTemplate.exchange(url, HttpMethod.GET, null, String.class);
// 处理成功响应
} catch (HttpServerErrorException e) {
if (e.getStatusCode() == HttpStatus.INTERNAL_SERVER_ERROR) {
// 处理 500 错误
} else {
// 处理其他 5xx 错误
}
}
```
3. ResourceAccessException:在网络连接或请求超时等异常情况下,会抛出此异常。可以通过捕获该异常来处理网络异常情况。
```java
try {
ResponseEntity<String> response = restTemplate.exchange(url, HttpMethod.GET, null, String.class);
// 处理成功响应
} catch (ResourceAccessException e) {
// 处理网络异常
}
```
除了上述异常处理,还可以通过使用 `ResponseErrorHandler` 接口来自定义异常处理。通过实现该接口,可以针对不同的响应状态码进行自定义处理。
```java
restTemplate.setErrorHandler(new DefaultResponseErrorHandler() {
@Override
public void handleError(ClientHttpResponse response) throws IOException {
if (response.getStatusCode() == HttpStatus.NOT_FOUND) {
// 处理 404 错误
} else if (response.getStatusCode() == HttpStatus.INTERNAL_SERVER_ERROR) {
// 处理 500 错误
} else {
super.handleError(response);
}
}
});
```
这样,在发起 HTTP 请求时,如果发生错误,就会根据自定义的异常处理进行处理。
阅读全文
相关推荐
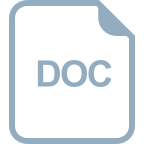
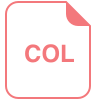






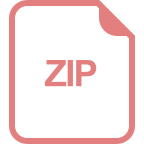
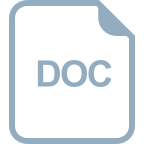






