python计算16位阈值分割后的图像中8邻接封闭区域面积
时间: 2024-09-28 12:13:16 浏览: 43
在Python中,你可以使用OpenCV (cv2) 和 numpy 这两个库来计算16位二值图像中每个8邻接封闭区域的面积。以下是基本步骤:
1. 首先,加载和读取16位图像(灰度或二值)。`cv2.imread()` 可能需要指定正确的颜色空间(例如,使用 `cv2.IMREAD_GRAYSCALE`),然后将图像转换为16位整数:
```python
import cv2
image = cv2.imread('your_image_path', cv2.IMREAD_GRAYSCALE)
image = image.astype(np.uint16)
```
2. 应用阈值分割,通常使用 `cv2.threshold()` 或者自定义阈值处理算法得到二值图像:
```python
_, binary_image = cv2.threshold(image, threshold_value, 255, cv2.THRESH_BINARY | cv2.THRESH_OTSU)
```
这里的 `threshold_value` 是用于设置阈值的数值,`otsu` 参数则自动选择最佳阈值。
3. 使用形态学操作消除小噪声并连接相邻的像素,这里可以使用膨胀(`cv2.dilate()`)和腐蚀(`cv2.erode()`):
```python
kernel = np.ones((3,3), np.uint8) # 生成3x3的结构元素
binary_image = cv2.morphologyEx(binary_image, cv2.MORPH_OPEN, kernel) # 扩大并收缩边缘
```
4. 寻找连通组件,也就是封闭区域,可以使用 `findContours()` 函数,它会返回轮廓数组:
```python
contours, _ = cv2.findContours(binary_image, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
```
注意 `_` 表示我们不需要内部轮廓。
5. 计算每个区域的面积,使用 `cv2.contourArea()`:
```python
areas = [cv2.contourArea(contour) for contour in contours]
```
最后,`areas` 列表包含了所有封闭区域的面积。
完整的代码示例:
```python
import cv2
import numpy as np
# ... (步骤1-3)
# Find and calculate areas of connected components
contours, hierarchy = cv2.findContours(binary_image, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
areas = [cv2.contourArea(contour) for contour in contours]
# Print or store the area values
for i, area in enumerate(areas):
print(f"Region {i+1}: Area = {area}")
```
阅读全文
相关推荐





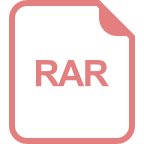










