vue3el-button怎么跳转到新的页面
时间: 2024-09-30 17:07:30 浏览: 61
在Vue3中,如果你想要使用`el-button`(Element Plus库中的按钮组件)实现页面之间的导航,你可以这样做:
首先,你需要安装Element Plus并引入Button组件。如果使用npm或yarn,可以在`main.js`或`setup.ts`文件中添加依赖:
```bash
npm install element-plus
# 或者
yarn add element-plus
```
然后在你的组件模板中,导入Button组件,并设置点击事件(通常会触发`router-link`或`nuxt-link`进行路由跳转,取决于你使用的路由解决方案,比如Vue Router或Nuxt.js):
```vue
<template>
<el-button @click="$router.push('/your-target-page')">跳转到新页面</el-button>
</template>
<script setup>
import { useRouter } from 'vue-router';
const router = useRouter();
</script>
```
在这里,`$router.push`是用来将用户导向新页面的Vue Router方法。替换`'/your-target-page'`为你要跳转的实际路径。
相关问题
vue 通过el-button使用组件跳转页面_vue.js 主页面组件替换或跳转
如果你使用了 Element UI 中的 `el-button` 组件,你可以通过在按钮点击事件中使用 Vue Router 实现页面跳转或组件替换的功能。以下是一个示例:
```vue
<template>
<div>
<el-button type="primary" @click="goToAnotherPage">跳转到另一个页面</el-button>
</div>
</template>
<script>
import { mapActions } from 'vuex';
export default {
methods: {
...mapActions(['goToAnotherPage']),
},
};
</script>
```
在上面的示例中,我们假设你已经按照之前提到的方式安装和配置了 Vue Router。然后,在按钮的点击事件中,调用 `goToAnotherPage` 方法实现页面跳转。
在路由配置文件中,你需要添加对应的路由规则,例如:
```javascript
import VueRouter from 'vue-router';
import AnotherPage from './components/AnotherPage.vue';
const routes = [
{ path: '/another-page', component: AnotherPage },
// 其他路由配置...
];
const router = new VueRouter({
routes,
});
export default router;
```
通过这种方式,当你点击按钮时,会触发路由跳转,从而替换或跳转到另一个页面组件。
记得在使用 `el-button` 组件之前,确保已经正确引入了 Element UI,并按照官方文档进行了正确的配置。
vue怎么用el-button跳转到另一个页面
可以使用`<router-link>`组件来实现跳转,具体操作如下:
1. 首先在`<template>`标签中,使用`<router-link>`组件,设定要跳转的路径,如下所示:
```html
<router-link to="/other-page">
<el-button>跳转到另一个页面</el-button>
</router-link>
```
2. 然后在 Vue 组件中,需要在`<script>`标签中引入`VueRouter`,并注册路由,在`routes`数组中添加要跳转的路由,如下所示:
```javascript
import VueRouter from 'vue-router'
import OtherPage from './OtherPage.vue'
const router = new VueRouter({
routes: [
{
path: '/other-page',
component: OtherPage
}
]
})
```
其中,`OtherPage`代表要跳转的页面组件,在这里需要在`<script>`标签中引入。
3. 最后,需要在 Vue 根实例中,将路由实例挂载到根实例上,如下所示:
```javascript
new Vue({
el: '#app',
router, // 将路由实例挂载到根实例上
components: { App },
template: '<App/>'
})
```
这样,当用户点击`<el-button>`按钮时,就会自动跳转到`/other-page`路径下的页面。
阅读全文
相关推荐
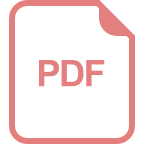
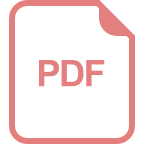
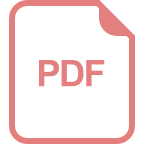














